用c++,写一个有栈结构的中缀表达式求值的代码
时间: 2024-05-07 17:20:17 浏览: 8
以下是一个基于栈实现的中缀表达式求值的 C++ 代码示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 计算两个数的结果
double calculate(double num2, double num1, char oper) {
switch (oper) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
return num1 / num2;
default:
return 0;
}
}
// 判断字符是否为操作符
bool isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
// 中缀表达式求值函数
double evaluateInfix(string expression) {
stack<double> numStack; // 存储数字的栈
stack<char> operStack; // 存储操作符的栈
for (int i = 0; i < expression.length(); i++) {
char c = expression[i];
// 如果当前字符是空格,直接忽略
if (isspace(c)) {
continue;
}
// 如果当前字符是数字,将其转换为实数并压入数字栈
if (isdigit(c)) {
double num = c - '0';
while (i + 1 < expression.length() && isdigit(expression[i + 1])) {
num = num * 10 + (expression[++i] - '0');
}
numStack.push(num);
}
// 如果当前字符是操作符,将其压入操作符栈
else if (isOperator(c)) {
while (!operStack.empty() && operStack.top() != '(' &&
((c != '*' && c != '/') || (operStack.top() != '+' && operStack.top() != '-'))) {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(calculate(num2, num1, operStack.top()));
operStack.pop();
}
operStack.push(c);
}
// 如果当前字符是左括号,将其压入操作符栈
else if (c == '(') {
operStack.push(c);
}
// 如果当前字符是右括号,不断弹出操作符栈顶的操作符并计算,直到遇到左括号为止
else if (c == ')') {
while (!operStack.empty() && operStack.top() != '(') {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(calculate(num2, num1, operStack.top()));
operStack.pop();
}
operStack.pop(); // 弹出左括号
}
}
// 将剩余的操作符计算完
while (!operStack.empty()) {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(calculate(num2, num1, operStack.top()));
operStack.pop();
}
return numStack.top(); // 返回最终结果
}
int main() {
string expression = "3 + 4 * (2 - 1)";
double result = evaluateInfix(expression);
cout << expression << " = " << result << endl;
return 0;
}
```
以上代码实现了一个简单的中缀表达式求值函数,其中使用了两个栈分别存储数字和操作符。在遍历表达式字符串时,对于不同类型的字符会进行不同的处理:
- 如果是数字,将其转换为实数并压入数字栈。
- 如果是操作符,从操作符栈中弹出相应的操作数,并进行计算,将结果压入数字栈。
- 如果是左括号,将其压入操作符栈。
- 如果是右括号,不断弹出操作符栈顶的操作符并计算,直到遇到左括号为止。
最终,在遍历完整个字符串后,将剩余的操作符依次计算即可得到最终结果。
相关推荐
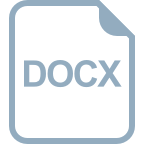














