用c语言创建一个搜索二叉树深度的代码片段
时间: 2024-10-24 09:02:44 浏览: 26
在 C 语言中,我们可以使用递归的方式来计算二叉搜索树(BST)的深度。下面是一个简单的代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} Node;
// 计算二叉树深度的辅助函数
int maxDepth(Node* root) {
if (root == NULL) // 如果根节点为空,则返回0
return 0;
// 分别计算左子树和右子树的最大深度,然后取较大值加1作为当前节点的深度
int left_depth = maxDepth(root->left);
int right_depth = maxDepth(root->right);
// 返回当前节点的深度,即左右子树最大深度之和
return left_depth > right_depth ? left_depth + 1 : right_depth + 1;
}
// 主函数演示如何使用
int main() {
// 初始化你的二叉搜索树...
// ...
Node* root = /* 替换为你的实际二叉搜索树的根节点 */;
int tree_depth = maxDepth(root);
printf("The depth of the binary search tree is %d\n", tree_depth);
return 0;
}
```
在这个代码片段中,`maxDepth` 函数递归地遍历二叉树直到找到空节点,同时记录并返回深度。记得在 `main` 函数里替换实际的二叉搜索树根节点。
阅读全文
相关推荐
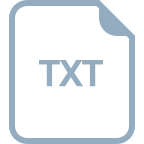
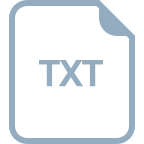
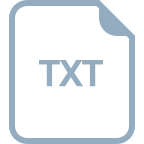
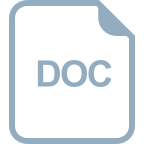

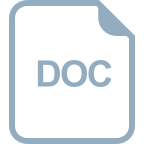
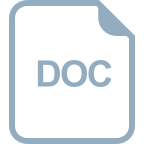
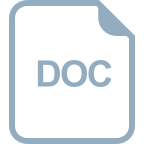



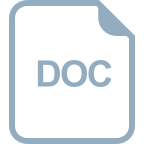
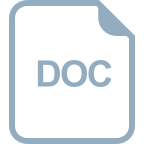
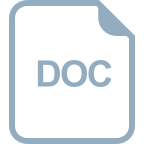
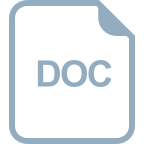
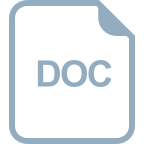

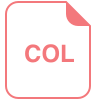

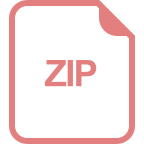