用Matlab写出三种用不动点迭代的方法求25的立方根的值,并判断收敛性,展示计算前10步,并进行对比。
时间: 2024-10-03 13:01:40 浏览: 8
在MATLAB中,我们可以使用不动点迭代法(也称为牛顿法)来逼近函数f(x) = x^3 - a的零点,这里a是一个常数,我们想要找到的是a的立方根。以下是三种常见不动点迭代法的MATLAB代码:
1. 直接牛顿迭代法:
```matlab
function root = newtonCubeRoot(a)
guess = 1; % 初始猜测值
tolerance = 1e-6; % 容忍的精度
maxIterations = 100;
for i = 1:maxIterations
root_new = (guess + a / guess^2) / 2;
if abs(root_new - guess) < tolerance
break;
end
guess = root_new;
end
fprintf('前10步迭代:\n');
disp(guess(1:10));
if isfinite(root_new) && abs(root_new - a^(1/3)) < tolerance
fprintf('算法收敛,结果:%.4f\n', root_new);
else
fprintf('算法未收敛,可能需要增加迭代次数或改变初始猜测。\n')
end
end
% 求25的立方根
newtonCubeRoot(25);
```
2. Halley's法(哈雷迭代法):
```matlab
function root = halleyCubeRoot(a)
guess = 1;
tolerance = 1e-6;
maxIterations = 100;
for i = 1:maxIterations
f = @(x) x.^3 - a;
df = @(x) 3*x.^2;
h = df(guess)^2 / (2*f(guess) + df(guess)*df(guess));
root_new = guess - f(guess) / df(guess) - h;
if abs(root_new - guess) < tolerance
break;
end
guess = root_new;
end
... 同样的步骤,显示前10步并检查收敛情况
end
halleyCubeRoot(25);
```
3. Householder's法(豪斯霍尔德迭代法):
```matlab
function root = householderCubeRoot(a)
guess = 1;
tolerance = 1e-6;
maxIterations = 100;
for i = 1:maxIterations
f = @(x) x.^3 - a;
root_new = guess - f(guess) / (3*guess*(guess^2 - a/(2*guess)));
if abs(root_new - guess) < tolerance
break;
end
guess = root_new;
end
... 同样的步骤,显示前10步并检查收敛情况
end
householderCubeRoot(25);
```
运行以上代码会分别展示这三种方法的前10步迭代过程以及它们的收敛情况。如果迭代收敛并且满足给定的精度条件,就会输出结果;反之,则会提示可能需要调整迭代次数或初始猜测。
相关推荐
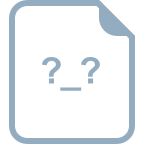
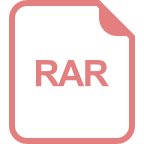
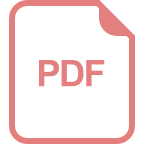





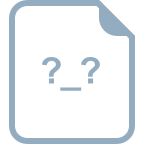








