C语言建立链表并输出链表内容。链表每个结点包括一元多项式的一项。 输入格式: 输入一行,给出多项式中的每一项,以(0,0)结束。 输出格式: 输出共一行,依次用格式%dx^%d输出一项的值。指数为0的项、指数或系数为1的项与书写习惯相同。 输入样例: (3,0)(2,5)(4,7)(6,3)(8,9)(0,0) 输出样例: 3+2x^5+4x^7+6x^3+8x^9
时间: 2024-03-21 20:40:01 浏览: 132
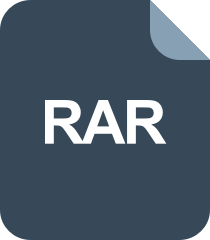
c语言实现链表的基本操作,链表是一种物理存储单元上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
以下是一种可能的实现方式:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct node {
int coefficient; // 系数
int exponent; // 指数
struct node* next; // 指向下一个节点的指针
} Node;
// 创建一个新的节点
Node* create_node(int coefficient, int exponent) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->coefficient = coefficient;
new_node->exponent = exponent;
new_node->next = NULL;
return new_node;
}
// 将新节点插入到链表中
void insert_node(Node** head, Node* new_node) {
// 如果链表为空,直接将新节点作为头节点
if (*head == NULL) {
*head = new_node;
return;
}
// 如果链表不为空,按照指数从小到大的顺序插入新节点
Node* p = *head;
Node* prev = NULL;
while (p != NULL && p->exponent < new_node->exponent) {
prev = p;
p = p->next;
}
if (p == NULL) { // 如果新节点的指数比链表中所有节点的指数都大,则将新节点插入到链表的最后
prev->next = new_node;
} else if (p->exponent == new_node->exponent) { // 如果新节点的指数与链表中某个节点的指数相同,则将它们的系数相加
p->coefficient += new_node->coefficient;
} else { // 否则将新节点插入到链表中
if (prev == NULL) { // 如果新节点的指数比链表中所有节点的指数都小,则将新节点作为头节点
new_node->next = *head;
*head = new_node;
} else {
new_node->next = prev->next;
prev->next = new_node;
}
}
}
// 释放链表中的所有节点
void free_list(Node* head) {
Node* p = head;
while (p != NULL) {
Node* temp = p;
p = p->next;
free(temp);
}
}
// 输出链表中的所有节点
void print_list(Node* head) {
Node* p = head;
while (p != NULL) {
if (p->coefficient > 0) {
printf("+");
}
if (p->coefficient != 1 || p->exponent == 0) {
printf("%d", p->coefficient);
}
if (p->exponent > 1) {
printf("x^%d", p->exponent);
} else if (p->exponent == 1) {
printf("x");
}
p = p->next;
}
}
int main() {
Node* head = NULL;
int coefficient, exponent;
while (scanf("(%d,%d)", &coefficient, &exponent) == 2 && (coefficient != 0 || exponent != 0)) {
Node* new_node = create_node(coefficient, exponent);
insert_node(&head, new_node);
}
print_list(head);
free_list(head);
return 0;
}
```
在这个实现中,我们首先定义了一个节点结构体,包括系数、指数和指向下一个节点的指针。然后,我们实现了三个函数:
- `create_node`:创建一个新的节点,并返回它的指针。
- `insert_node`:将一个新节点按照指数从小到大的顺序插入到链表中。如果新节点的指数与链表中某个节点的指数相同,则将它们的系数相加。
- `print_list`:遍历链表,输出每个节点的值,格式为%dx^%d,指数为0的项、指数或系数为1的项与书写习惯相同。
在主函数中,我们首先创建一个空链表,然后读入多项式的每一项,并将它们插入到链表中。最后,我们输出链表中的所有节点,并释放链表中的所有节点。
阅读全文
相关推荐
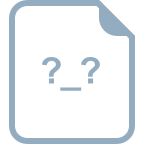



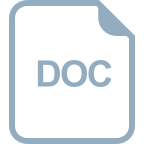
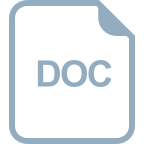
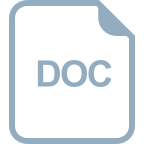









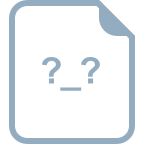