用python模拟退火算法
时间: 2024-06-19 22:00:56 浏览: 9
Python模拟退火算法是一种用于求解优化问题的搜索方法,它模仿了金属冷却过程中的相变行为。该算法主要用于解决全局优化问题,比如寻找函数的最小值。以下是使用Python实现模拟退火的基本步骤:
1. 初始化:选择一个随机解作为初始状态,并设置初始温度(T),冷却速率(alpha)和接受阈值(acceptance probability)。
2. 计算能量:计算当前解的“能量”或目标函数值。能量越低,解越接近最优。
3. 探索邻域:从当前解生成一个邻近解,可能是随机的微小变化。
4. 比较能量:计算新解的能量。如果新解能量更低,接受;如果更高,根据一定的概率接受,这个概率由当前温度决定,遵循Metropolis准则。
5. 温度更新:根据接受率和预设的冷却速率,降低温度。当温度低于某个阈值(如0)或达到迭代次数限制,停止探索。
6. 返回结果:记录下最低能量的解作为最终结果。
以下是一个简单的Python模拟退火算法的代码示例:
```python
import random
def func(x):
# 这里假设func是你要优化的目标函数
return x**2
def random_neighbor(current_state, temp):
# 生成随机邻域
new_state = current_state + random.uniform(-1, 1)
return new_state if random.random() < temp else current_state
def simulated_annealing(start_state, T=1000, alpha=0.99, max_iterations=1000):
current_state = start_state
current_energy = func(current_state)
best_state = current_state
best_energy = current_energy
for _ in range(max_iterations):
new_state = random_neighbor(current_state, T)
new_energy = func(new_state)
if new_energy < current_energy or random.random() < min(1, math.exp(-(new_energy - current_energy) / T)):
current_state = new_state
current_energy = new_energy
if new_energy < best_energy:
best_state = current_state
best_energy = new_energy
T *= alpha
if T < 1e-6:
break
return best_state, best_energy
# 示例
start_state = [random.uniform(-100, 100) for _ in range(10)] # 假设你有一个10维的优化问题
best_state, best_energy = simulated_annealing(start_state)
print(f"最优解: {best_state}, 最低能量: {best_energy}")
```
相关推荐
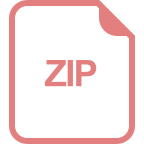













