c++设计复数类,实现复数的+、-、*、
时间: 2024-10-10 20:11:10 浏览: 25
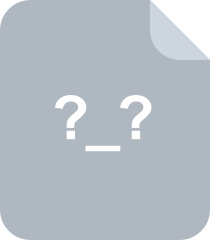
C++程序, 设计复数类

在C++中设计一个复数类(Complex),我们可以使用结构体或者类来实现,这里以类为例。复数由实部(real)和虚部(imaginary)组成,可以实现加法、减法、乘法操作。下面是一个简单的复数类实现:
```cpp
#include <iostream>
class Complex {
private:
double real; // 实部
double imaginary; // 虚部
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imaginary(i) {}
// 拷贝构造函数和赋值运算符为了防止浅拷贝,增加深拷贝
Complex(const Complex& other) : real(other.real), imaginary(other.imaginary) {}
Complex& operator=(const Complex& other) {
if (this != &other) { // 防止自赋值
real = other.real;
imaginary = other.imaginary;
}
return *this;
}
// 加法
Complex operator+(const Complex& b) const {
return Complex(real + b.real, imaginary + b.imaginary);
}
// 减法
Complex operator-(const Complex& b) const {
return Complex(real - b.real, imaginary - b.imaginary);
}
// 乘法
Complex operator*(const Complex& b) const {
double newReal = real * b.real - imaginary * b.imaginary;
double newImaginary = real * b.imaginary + imaginary * b.real;
return Complex(newReal, newImaginary);
}
// 打印复数
void print() const {
std::cout << real << " + " << imaginary << "i" << std::endl;
}
};
int main() {
Complex a(3, 4);
Complex b(1, 2);
a.print(); // 输出 3 + 4i
Complex sum = a + b;
sum.print(); // 输出 4 + 6i
Complex product = a * b;
product.print(); // 输出 5 + 10i
return 0;
}
```
在这个例子中,我们定义了基本的操作以及必要的复制控制。
阅读全文
相关推荐
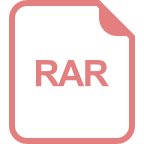
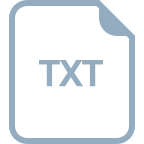
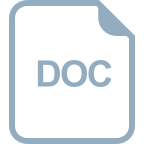


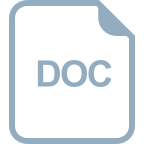





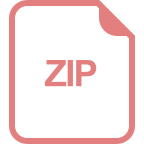





