能否提供一个C语言编程示例,展示如何将中缀表达式转换为对应的波兰(后缀)表达式算法?
时间: 2024-11-05 14:33:08 浏览: 32
当然可以。在C语言中,我们可以使用递归的方式来实现中缀表达式到后缀表达式的转换。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h> // 用于字符分类
// 常量定义运算符优先级
#define LEFT_PAREN 0
#define ADD_SUB 1
#define MUL_DIV 2
#define MAX_PRECEDENCE 3
// 函数声明
char *convertInfixToPostfix(char *infix, char *postfix);
// 助手函数,获取下一个需要处理的字符
char getNextChar(char *str) {
while (*str == ' ' || *str == '\t' || *str == '\n') str++;
return *str;
}
// 判断字符是否为运算符
int isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
// 助手函数,处理运算符并返回它的优先级
int getPriority(char c) {
switch (c) {
case '+':
case '-': return ADD_SUB;
case '*':
case '/': return MUL_DIV;
default: return MAX_PRECEDENCE; // 非运算符视为最高优先级
}
}
// 主函数
char *convertInfixToPostfix(char *infix, char *postfix) {
char *out = postfix;
char next = getNextChar(infix);
// 遍历整个中缀表达式
while (next != '\0') {
// 如果是数字,直接复制到后缀表达式
if (isdigit(next)) {
while (isdigit(next)) {
*out++ = next;
next = getNextChar(infix);
}
*out++ = '\0';
} else if (next == '(') { // 处理左括号
out = appendToPostfix(out, next, postfix);
next = getNextChar(infix);
} else if (next == ')') { // 处理右括号
out = appendToPostfix(out, ')', postfix);
next = getNextChar(infix);
} else if (isOperator(next)) { // 处理运算符
out = appendToPostfix(out, next, postfix);
while (getNextChar(infix) != '\0' && getPriority(getNextChar(infix)) == getPriority(next))
out = appendToPostfix(out, getNextChar(infix), postfix);
next = getNextChar(infix);
}
}
return postfix;
}
// 助手函数,将字符追加到后缀表达式中,并处理优先级嵌套
char *appendToPostfix(char *dest, char op, char *postfix) {
*dest++ = op;
while (postfix != NULL && postfix[0] == '(')
dest = appendToPostfix(dest, ')', postfix);
return dest;
}
// 测试
int main() {
char infix[30], postfix[100];
fgets(infix, sizeof(infix), stdin);
postfix = convertInfixToPostfix(infix, postfix);
printf("后缀表达式: %s\n", postfix);
return 0;
}
```
在这个示例中,我们首先遍历输入的中缀表达式,识别每个字符是数字、运算符还是括号。然后,根据运算符的优先级规则,将它们添加到后缀表达式中。括号会临时保存,直到遇到更低优先级的运算符或结束。
阅读全文
相关推荐









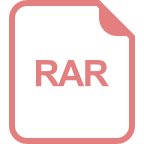
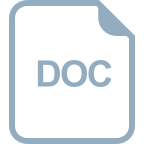
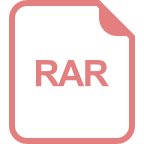






