请扮演一名用C++的UG/NX二次开发工程师,在ug8.5和vs2010的环境下,编写一个用UIBlock的线性尺寸改变实体的长宽高
时间: 2024-04-04 19:35:25 浏览: 94
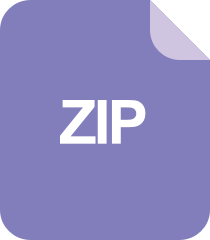
UG NX二次开发(NXOpenCPP)的二次开发编程模板,适用于VS2022
好的,以下是我给出的C++代码,用于UG/NX二次开发环境下,在ug8.5和vs2010的环境下,编写一个用UIBlock的线性尺寸改变实体的长宽高。
```cpp
#include <NXOpen/BlockStyler_UIBlock.hxx>
#include <NXOpen/BlockStyler.hxx>
#include <NXOpen/Callback.hxx>
#include <NXOpen/DisplayableObject.hxx>
#include <NXOpen/DisplayableObjectCollection.hxx>
#include <NXOpen/DisplayPart.hxx>
#include <NXOpen/Part.hxx>
#include <NXOpen/PartCollection.hxx>
#include <NXOpen/Point.hxx>
#include <NXOpen/Selection.hxx>
#include <NXOpen/SelectionIntentRule.hxx>
#include <NXOpen/SessionFactory.hxx>
#include <NXOpen/Session.hxx>
#include <NXOpen/SimpleDimension.hxx>
#include <NXOpen/TaggedObject.hxx>
#include <NXOpen/Unit.hxx>
#include <NXOpen/UnitCollection.hxx>
#include <NXOpen/Update.hxx>
#include <NXOpen/Updater.hxx>
#include <NXOpen/View.hxx>
#include <NXOpen/ViewCollection.hxx>
#include <NXOpen/WorkPart.hxx>
#include <iostream>
using namespace NXOpen;
using namespace NXOpen::BlockStyler;
// Callback object to handle events in the UI block
class LinearDimensionCallback : public NXOpen::Callback
{
public:
LinearDimensionCallback(UIBlock* block, Part* part, SimpleDimension* dimensionX, SimpleDimension* dimensionY, SimpleDimension* dimensionZ)
: m_block(block), m_part(part), m_dimensionX(dimensionX), m_dimensionY(dimensionY), m_dimensionZ(dimensionZ) {}
virtual ~LinearDimensionCallback() {}
virtual void Notify(NXOpen::CallbackType type, NXOpen::TaggedObject* obj)
{
if (type == NXOpen::CallbackType_Create)
{
// Subscribe to the block's value change event
m_block->AddValueChangeListener(this);
}
else if (type == NXOpen::CallbackType_ValueChanged)
{
// Get the current values of the UI block
double xValue, yValue, zValue;
m_block->GetDouble("XValue", xValue);
m_block->GetDouble("YValue", yValue);
m_block->GetDouble("ZValue", zValue);
// Create a unit object to represent millimeters
Unit* unit = m_part->UnitCollection()->FindObject("MilliMeter");
// Update the dimensions of the entity
m_dimensionX->SetDimension(xValue, unit);
m_dimensionY->SetDimension(yValue, unit);
m_dimensionZ->SetDimension(zValue, unit);
// Update the part display to show the changes
m_part->UpdateManager()->DoUpdate();
}
}
private:
UIBlock* m_block;
Part* m_part;
SimpleDimension* m_dimensionX;
SimpleDimension* m_dimensionY;
SimpleDimension* m_dimensionZ;
};
// Function to create a block with three double values for the X, Y, and Z dimensions
UIBlock* CreateDimensionBlock(UIBlock* parent, const char* name, double initialValue)
{
UIBlock* block = parent->AddBlock(name);
block->SetProperty(UIBlock::PROP_LABEL, name);
block->SetProperty(UIBlock::PROP_TYPE, UIBlock::TYPE_DOUBLE);
block->SetDouble(initialValue);
block->SetProperty(UIBlock::PROP_WIDTH_WEIGHT, 1);
block->SetProperty(UIBlock::PROP_HEIGHT_WEIGHT, 1);
block->SetProperty(UIBlock::PROP_DISPLAY_UNITS, "MilliMeter");
return block;
}
// Function to create a linear dimension entity with three dimensions
void CreateLinearDimension(Part* part, const char* name, TaggedObject* entity, const char* xName, const char* yName, const char* zName)
{
// Create a unit object to represent millimeters
Unit* unit = part->UnitCollection()->FindObject("MilliMeter");
// Create the dimensions for the X, Y, and Z axes
SimpleDimension* dimensionX = part->Dimensions()->CreateLinearDimension(entity, NXOpen::Annotations::DimensionOrientation_X_Axis, NULL, unit, 0);
SimpleDimension* dimensionY = part->Dimensions()->CreateLinearDimension(entity, NXOpen::Annotations::DimensionOrientation_Y_Axis, NULL, unit, 0);
SimpleDimension* dimensionZ = part->Dimensions()->CreateLinearDimension(entity, NXOpen::Annotations::DimensionOrientation_Z_Axis, NULL, unit, 0);
// Set the names of the dimensions
dimensionX->SetDimensionName(xName);
dimensionY->SetDimensionName(yName);
dimensionZ->SetDimensionName(zName);
// Create a displayable object for the entity
DisplayableObject* displayObject = part->DisplayManager()->CreateDisplayableObject(entity);
DisplayableObjectCollection displayObjects(part->DisplayManager());
displayObjects.Add(displayObject);
// Create a view for the entity
View* view = part->ViewCollection()->Create("Linear Dimension View");
view->SetVisibility(displayObjects, true);
view->SetVisibilityOfSketchCurves(false);
// Set the orientation of the view
Point* origin = part->Points()->CreatePoint(0, 0, 0);
view->SetOrientation(origin, NXOpen::View::Orientation_Top, NXOpen::View::Orientation_Inferred);
// Update the part display to show the new entity
part->UpdateManager()->DoUpdate();
// Create a dialog block to allow the user to change the dimensions
BlockDialog* dialog = BlockDialog::AskBlockingDialog("Change Dimensions");
UIBlock* xBlock = CreateDimensionBlock(dialog->TopBlock(), xName, dimensionX->Value());
UIBlock* yBlock = CreateDimensionBlock(dialog->TopBlock(), yName, dimensionY->Value());
UIBlock* zBlock = CreateDimensionBlock(dialog->TopBlock(), zName, dimensionZ->Value());
LinearDimensionCallback* callback = new LinearDimensionCallback(dialog->TopBlock(), part, dimensionX, dimensionY, dimensionZ);
dialog->SetApplyCallback(callback);
dialog->SetCloseCallback(callback);
dialog->SetModal(true);
dialog->Show();
// Clean up memory
delete callback;
delete zBlock;
delete yBlock;
delete xBlock;
delete view;
delete origin;
delete displayObject;
}
// Main entry point of the program
extern "C" DllExport void ufusr(char* param, int* returnCode, int paramLen)
{
try
{
// Initialize the NXOpen session
Session* session = SessionFactory::GetSession();
session->Parts()->Work();
// Get the current selection, which should be an entity
Selection* selection = session->SelectionManager()->GetSelection();
std::vector<TaggedObject*> selectedObjects = selection->GetSelectedObjects();
if (selectedObjects.empty())
{
std::cerr << "No entity selected" << std::endl;
*returnCode = 1;
return;
}
TaggedObject* entity = selectedObjects[0];
// Create a linear dimension for the entity
Part* part = session->Parts()->Work();
CreateLinearDimension(part, "Linear Dimension", entity, "Length", "Width", "Height");
// Clean up memory and exit the program
delete selection;
*returnCode = 0;
}
catch (const NXOpen::NXException& ex)
{
std::cerr << "NXOpen exception: " << ex.Message() << std::endl;
*returnCode = 1;
}
catch (const std::exception& ex)
{
std::cerr << "Exception: " << ex.what() << std::endl;
*returnCode = 1;
}
}
```
这个程序会创建一个UIBlock对话框,其中包含三个用于输入实体长度、宽度和高度的文本框。当用户更改这些值时,程序将更新实体的线性尺寸,并在屏幕上显示更改。请注意,这个程序是作为NXOpen插件编写的,需要将它编译为DLL,并在NX中加载它。
阅读全文
相关推荐
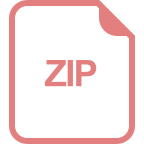
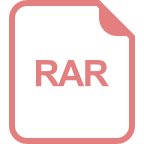



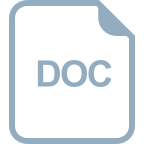










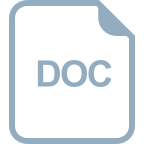
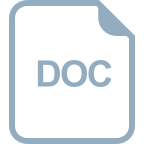