unity第三人称人物移动
时间: 2023-09-25 12:07:32 浏览: 26
在Unity中,第三人称人物移动可以使用CharacterController组件。首先需要将角色模型添加到场景中,并附加CharacterController组件。接下来可以使用Input.GetAxis函数获取玩家输入的移动方向并乘以移动速度,最后使用CharacterController.Move函数实现移动。如果需要更加精细的控制,可以使用刚体组件和力来实现。
相关问题
Unity第三人称移动
以下是一个简单的第三人称移动示例,包括人物的移动、转向和动画播放:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ThirdPersonMovement : MonoBehaviour
{
public CharacterController controller;
public Transform cam;
public Animator animator;
public float speed = 6f;
public float turnSmoothTime = 0.1f;
float turnSmoothVelocity;
// Update is called once per frame
void Update()
{
float horizontal = Input.GetAxisRaw("Horizontal");
float vertical = Input.GetAxisRaw("Vertical");
Vector3 direction = new Vector3(horizontal, 0f, vertical).normalized;
if (direction.magnitude >= 0.1f)
{
float targetAngle = Mathf.Atan2(direction.x, direction.z) * Mathf.Rad2Deg + cam.eulerAngles.y;
float angle = Mathf.SmoothDampAngle(transform.eulerAngles.y, targetAngle, ref turnSmoothVelocity, turnSmoothTime);
transform.rotation = Quaternion.Euler(0f, angle, 0f);
Vector3 moveDir = Quaternion.Euler(0f, targetAngle, 0f) * Vector3.forward;
controller.Move(moveDir.normalized * speed * Time.deltaTime);
animator.SetBool("isWalking", true);
}
else
{
animator.SetBool("isWalking", false);
}
}
}
```
其中,CharacterController是Unity提供的用于角色控制的组件,cam是摄像机的Transform组件,animator是角色的动画控制器。在Update函数中,首先获取水平和垂直方向上的输入值,然后计算出移动方向并进行平滑转向,最后移动角色并播放相应的动画。
unity第一人称视角移动
在 Unity 中实现第一人称视角移动可以通过以下步骤:
1. 创建一个空的 GameObject 并将其命名为 Player。
2. 在 Player 对象下创建一个 Camera 子对象,并将其命名为 MainCamera。
3. 将 MainCamera 对象的 Position 设置在 Player 对象的前方,使其成为第一人称视角。
4. 在 Player 对象上添加一个 CharacterController 组件,用于控制角色的移动。
5. 编写代码控制角色的移动,例如:
```csharp
public class PlayerController : MonoBehaviour
{
public float speed = 6.0f;
private CharacterController controller;
private Vector3 moveDirection = Vector3.zero;
void Start()
{
controller = GetComponent<CharacterController>();
}
void Update()
{
// 获取玩家的输入
float h = Input.GetAxis("Horizontal");
float v = Input.GetAxis("Vertical");
// 计算移动方向
Vector3 forward = transform.TransformDirection(Vector3.forward);
Vector3 right = transform.TransformDirection(Vector3.right);
moveDirection = (h * right + v * forward).normalized;
// 应用移动
moveDirection *= speed;
controller.Move(moveDirection * Time.deltaTime);
}
}
```
以上代码实现了通过键盘输入控制角色在水平方向和垂直方向上的移动,移动速度为6.0f。可以根据需要进行修改。
相关推荐
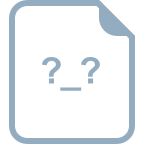












