用c语言实现在一个非递减有序数列中,插入一个值为x的元素,使插入后的链表仍为非递减排序
时间: 2024-10-20 07:02:38 浏览: 21
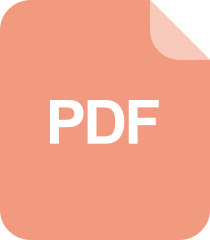
探讨:将两个链表非降序合并为一个链表并依然有序的实现方法
在C语言中,要在非递减有序链表中插入一个值为x的新节点,你需要创建一个新的节点并将其正确地插入到已排序链表中。这里是一个基本步骤:
1. 创建新节点:首先,定义一个新的节点结构体,通常包含一个整数值`data`和两个指针,一个指向当前节点`next`,另一个用于前驱节点`prev`(如果插入位置不是头节点)。
```c
typedef struct Node {
int data;
struct Node* next;
struct Node* prev;
} Node;
```
2. 检查链表是否为空:如果链表的第一个节点(head)是NULL,那么直接将新节点设为头节点。
3. 遍历链表:找到插入位置。遍历过程中,如果你发现当前节点的值大于等于x,说明应该在这之后插入。
4. 插入节点:创建新节点,并设置其数据为x。然后根据链表的状态更新指针连接。如果是头节点,设置`new_node->next = head`;否则,`new_node->prev = current_node` 和 `current_node->next = new_node`。
5. 如果链表已经完全按照非递减顺序排列,而x比所有节点都大,只需将x设为最后一个节点的下一个位置即可,`last_node->next = new_node`。
以下是伪代码形式:
```c
void insert_sorted(Node** head, int x) {
Node* new_node = malloc(sizeof(Node));
new_node->data = x;
if (*head == NULL || (*head)->data >= x) {
// 将新节点作为新的头节点
new_node->prev = NULL;
if (*head != NULL)
(*head)->prev = new_node;
*head = new_node;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data < x) {
current = current->next;
}
// 更新指针,根据找到的位置插入
new_node->prev = current;
if (current->next != NULL)
current->next->prev = new_node;
new_node->next = current->next;
current->next = new_node;
}
}
```
阅读全文
相关推荐
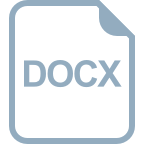
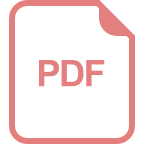















