edges = G.edges();如果是这样编程的话,会产生Python 错误 TypeError: 'cached_property' object is not callable
时间: 2024-09-25 16:03:37 浏览: 18
当你看到这样的错误 `TypeError: 'cached_property' object is not callable`,这通常意味着你在尝试像普通函数一样调用一个属性,但实际上它是一个缓存装饰器(cached_property)的结果。`edges` 是 NetworkX(一种常用的Python图形处理库)中的一个特性,它应该是返回一个边集合的迭代器,而不是可以直接调用的函数。
NetworkX的 `G.edges()` 返回的是一个生成器对象,你可以遍历它来查看图的所有边,而不是直接得到一个列表。例如:
```python
# 如果G是一个网络x
for edge in edges:
u, v = edge
print(f"Edge between {u} and {v}")
```
如果你想一次性获取所有的边,你应该这样做:
```python
all_edges = list(G.edges()) # 这会生成并储存所有边到list里
```
如果你试图对`edges`执行其他非迭代操作,如计算长度、过滤等,那就会触发错误,因为它是不可调用的。记得缓存装饰器的作用是提高性能,通过记住结果只计算一次,而不是作为函数处理输入。
相关问题
请解释以下代码:edges_unordered = np.genfromtxt(path, dtype=np.int32) edges = np.array(list(map(idx_map.get, edges_unordered.flatten())), dtype=np.int32).reshape(edges_unordered.shape) adj = sp.coo_matrix((np.ones(edges.shape[0]), (edges[:, 0], edges[:, 1])), shape=(n, n), dtype=np.float32)
这段代码的功能是将一个存储有无序边的文件读入,并将其转换为一个稀疏的邻接矩阵。
具体地,该代码的执行过程如下:
1. 使用NumPy的`genfromtxt`函数从指定路径的文件中读入数据,数据类型为32位整数数组,赋值给变量`edges_unordered`。
2. 将`edges_unordered`数组展平成一维数组,并使用`map`函数对每个节点编号进行映射,将其转换为对应的索引值。映射关系保存在`idx_map`字典中。将映射后的一维数组转换为32位整数数组,并按照`edges_unordered`的形状重新reshape为二维数组,赋值给变量`edges`。
3. 利用NumPy的`ones`函数生成一个长度为`edges.shape[0]`的一维数组,元素值全为1。使用`edges`数组中的节点索引和该一维数组中的1值创建一个稀疏的COO格式矩阵,表示邻接矩阵。该矩阵的形状为`(n, n)`,数据类型为32位浮点数,赋值给变量`adj`。
最终,`adj`就是一个表示无向图邻接矩阵的稀疏矩阵,其中每个元素表示两个节点之间是否存在一条边。
import networkx as nx # 创建一个有向图 G = nx.DiGraph() # 添加边 for index, row in df.iterrows(): G.add_edge(row['尾节点'], row['头节点'], length=row['长度'], capacity=row['容量']) # 计算所有节点之间的最短路径 shortest_paths = dict(nx.floyd_warshall(G, weight='length')) # 获取起始节点和目标节点之间的最短路径 path = nx.shortest_path(G, source='起始节点', target='目标节点', weight='length')# 初始化每条边的使用次数为0 edge_count = {} for index, row in df.iterrows(): edge_count[(row['尾节点'], row['头节点'])] = 0 # 计算每条边被使用的次数 for i in range(len(path)-1): edge_count[(path[i], path[i+1])] += 1 # 按照使用次数对所有边进行排序 sorted_edges = sorted(edge_count.items(), key=lambda x: x[1], reverse=True) # 显示排序结果 for edge, count in sorted_edges: print(edge, count)报错TypeError: 'module' object is not callable,如何解决
这个错误可能是因为你的代码中使用了与模块同名的变量或函数名。
首先,确保你已经正确导入了 `networkx` 模块。如果你的代码中有同名的变量或函数,可以修改变量名或函数名,避免与模块名相同。
如果以上方法都不行,可以尝试使用完整的模块名称 `nx.shortest_path` 来调用函数,例如:
```
path = nx.shortest_path(G, source='起始节点', target='目标节点', weight='length')
```
另外,你的代码中使用了 `nx.floyd_warshall` 函数来计算所有节点之间的最短路径,因此你可以使用 `shortest_paths` 字典来获取节点之间的最短路径,而不是使用 `nx.shortest_path` 函数。例如:
```
path = shortest_paths['起始节点']['目标节点']
```
最后,你的代码中使用了 `edge_count.items()` 来获取边的使用次数,但是 `edge_count` 是一个字典,应该使用 `edge_count.items()`。例如:
```
sorted_edges = sorted(edge_count.items(), key=lambda x: x[1], reverse=True)
```
如果还有问题,请提供更多的错误信息和代码上下文,以便更好地解决问题。
阅读全文
相关推荐
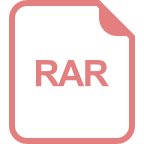
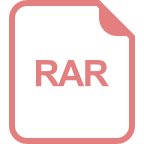














