3.(1)设计平面坐标点(Point)类,计算两点之间距离、到原点距离、关于坐标轴的对称点。(2)建立点(3,5)和点(-2,4),计算它们之间距离、到原点距离、关于坐标轴的对称点。
时间: 2023-11-22 12:51:45 浏览: 25
(1) Point类代码如下:
```python
import math
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def distance(self, other):
return math.sqrt((self.x - other.x) ** 2 + (self.y - other.y) ** 2)
def distance_to_origin(self):
return math.sqrt(self.x ** 2 + self.y ** 2)
def reflect(self):
return Point(-self.x, -self.y)
```
其中,`__init__`方法初始化点的坐标,`distance`方法计算两点之间的距离,`distance_to_origin`方法计算点到原点的距离,`reflect`方法计算点关于坐标轴的对称点。
(2) 建立点(3,5)和点(-2,4),计算它们之间距离、到原点距离、关于坐标轴的对称点的代码如下:
```python
point1 = Point(3, 5)
point2 = Point(-2, 4)
print("The distance between point1 and point2 is:", point1.distance(point2))
print("The distance from point1 to origin is:", point1.distance_to_origin())
print("The distance from point2 to origin is:", point2.distance_to_origin())
reflected_point1 = point1.reflect()
reflected_point2 = point2.reflect()
print("The reflection of point1 is:", reflected_point1.x, reflected_point1.y)
print("The reflection of point2 is:", reflected_point2.x, reflected_point2.y)
```
输出结果为:
```
The distance between point1 and point2 is: 5.0990195135927845
The distance from point1 to origin is: 5.830951894845301
The distance from point2 to origin is: 4.47213595499958
The reflection of point1 is: -3 -5
The reflection of point2 is: 2 -4
```
可以看到,点(3, 5)和点(-2, 4)之间的距离为5.0990,点(3, 5)到原点的距离为5.8309,点(-2, 4)到原点的距离为4.4721。点(3, 5)关于坐标轴的对称点为(-3, -5),点(-2, 4)关于坐标轴的对称点为(2, -4)。
相关推荐
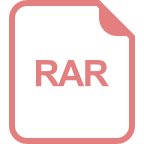
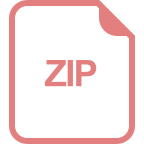











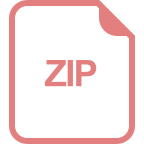
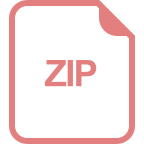