out = torch.cat(TT, dim=1)
时间: 2023-09-12 12:12:18 浏览: 59
`torch.cat(TT, dim=1)`是将一个列表 `TT` 中的张量在指定维度上进行拼接的操作。
`torch.cat()`函数用于在指定维度上将多个张量拼接在一起。在这里,`TT` 是一个包含多个张量的列表,`dim=1` 表示在第1维(即列维度)上进行拼接。
具体来说,假设 `TT` 是一个包含3个张量的列表,每个张量的形状为 `(N, C, H, W)`,其中 `N` 是样本数量,`C` 是通道数,`H` 是高度,`W` 是宽度。那么 `torch.cat(TT, dim=1)` 将会按照列维度拼接这3个张量,形成一个新的张量,其形状为 `(N, 3C, H, W)`。
例如,如果 `TT` 包含以下3个张量:
```
TT = [tensor1, tensor2, tensor3]
```
其中 `tensor1.shape = (N, C, H, W)`, `tensor2.shape = (N, C, H, W)`, `tensor3.shape = (N, C, H, W)`。那么 `torch.cat(TT, dim=1)` 将返回一个新的张量 `out`,其形状为 `(N, 3C, H, W)`。
需要注意的是,拼接时要求非拼接维度的其他维度尺寸必须一致,否则会抛出异常。在这个例子中,拼接的是列维度,因此要求张量在其他维度上的尺寸必须相同才能成功拼接。
相关问题
def forward(self, states: torch.FloatTensor): out1 = torch.relu(self.fc1(states)) out2 = torch.relu(self.fc2(states)) out = torch.relu(self.fc3(torch.cat([out1, out2], dim=-1))) out = torch.relu(self.fc4(out)) out = self.fc_out(out) return out
这是一个前向传播方法,用于对输入的状态数据进行前向计算并返回输出结果。假设这个方法是定义在一个神经网络模型类中的。
1. `states: torch.FloatTensor`:这个方法接受一个`torch.FloatTensor`类型的输入`states`,表示输入的状态数据。
2. `out1 = torch.relu(self.fc1(states))`:通过全连接层`self.fc1`对输入`states`进行线性变换,并应用ReLU激活函数得到`out1`。
3. `out2 = torch.relu(self.fc2(states))`:通过全连接层`self.fc2`对输入`states`进行线性变换,并应用ReLU激活函数得到`out2`。
4. `out = torch.relu(self.fc3(torch.cat([out1, out2], dim=-1)))`:将`out1`和`out2`在最后一个维度上进行拼接,然后通过全连接层`self.fc3`进行线性变换,并应用ReLU激活函数得到`out`。
5. `out = torch.relu(self.fc4(out))`:通过全连接层`self.fc4`对`out`进行线性变换,并应用ReLU激活函数得到新的`out`。
6. `out = self.fc_out(out)`:通过最后一个全连接层`self.fc_out`对`out`进行线性变换,得到最终的输出结果。
7. `return out`:返回输出结果。
这个方法描述了一个神经网络模型中的前向计算过程,其中包括了多个全连接层和ReLU激活函数的应用。通过这些计算,模型可以将输入的状态数据映射为输出结果。
class GAT(nn.Module): def __init__(self, in_dim, out_dim, num_heads): super(GAT, self).__init__() self.num_heads = num_heads self.attentions = nn.ModuleList([nn.Linear(in_dim, out_dim) for _ in range(num_heads)]) self.out_att = nn.Linear(in_dim*num_heads, out_dim) def forward(self, x, adj): x = x.unsqueeze(1) x = x.transpose(2,0) x = torch.cat([att(x) for att in self.attentions], dim=1) alpha = F.softmax(torch.matmul(x, x.transpose(1, 2)) / self.num_heads, dim=-1) alpha = torch.where(alpha>0, alpha, torch.zeros_like(alpha)) # alpha = torch.where(adj.unsqueeze(-1).bool(), alpha, torch.zeros_like(alpha)) alpha = alpha / alpha.sum(dim=-2, keepdim=True) out = torch.matmul(alpha, x).squeeze(1) out = F.elu(self.out_att(out)) return out 这段代码中out的形状为(192,512),而self.out_att只能接受(128,512)的输入,这段代码应该怎么调整呢。我尝试在self部分增加一个线性全连接层linear(512,128),但是报错缺少必要的位置参数,我应该怎么办呢。这是pytorch版本
可以在 `forward` 函数中增加一个线性全连接层,将 `out` 的形状从(192,512)变为(192,128),代码如下:
```
class GAT(nn.Module):
def __init__(self, in_dim, out_dim, num_heads):
super(GAT, self).__init__()
self.num_heads = num_heads
self.attentions = nn.ModuleList([nn.Linear(in_dim, out_dim) for _ in range(num_heads)])
self.out_att = nn.Linear(in_dim*num_heads, out_dim)
self.linear = nn.Linear(512, 128) # 新增的全连接层
def forward(self, x, adj):
x = x.unsqueeze(1)
x = x.transpose(2,0)
x = torch.cat([att(x) for att in self.attentions], dim=1)
alpha = F.softmax(torch.matmul(x, x.transpose(1, 2)) / self.num_heads, dim=-1)
alpha = torch.where(alpha>0, alpha, torch.zeros_like(alpha))
# alpha = torch.where(adj.unsqueeze(-1).bool(), alpha, torch.zeros_like(alpha))
alpha = alpha / alpha.sum(dim=-2, keepdim=True)
out = torch.matmul(alpha, x).squeeze(1)
out = F.elu(self.out_att(out))
out = self.linear(out) # 新增的全连接层
return out
```
关于报错缺少必要的位置参数,可以检查一下代码中是否存在遗漏的参数或者参数位置错误的情况。如果还有问题可以提供更详细的错误信息以及代码段。
相关推荐
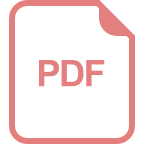
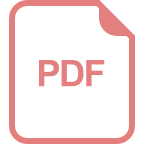













