用c语言求解二元一次方程的根
时间: 2024-11-09 22:18:10 浏览: 18
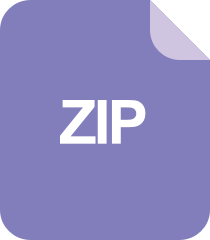
c语言求解二元二次方程问题
在C语言中,解决二元一次方程组通常涉及使用一些数学方法,比如代数法或数值方法(如高斯消元法或迭代法)。这里我们简单介绍如何通过高斯消元法来解形如ax + by = c 和 dx + ey = f 的线性方程组。
```c
#include <stdio.h>
#include <math.h>
// Function to perform row operations in matrix (Gaussian Elimination)
void gauss_elimination(float a[2][2], float b[2]) {
// Step 1: Check if the coefficient of x is non-zero
if (a[0][0] == 0) {
printf("Invalid equation system: Coefficient of x cannot be zero.\n");
return;
}
// Step 2: Divide first row by 'a[0][0]' for normalization
b[0] /= a[0][0];
a[1][0] /= a[0][0]; // Modify the second row accordingly
// Step 3: Subtract appropriate multiple of first row from second row to eliminate 'x' term
b[1] -= a[1][0] * b[0];
// Step 4: If determinant is zero, it means there's no unique solution
if (fabs(a[1][1]) < 1e-6) {
printf("Infinite solutions or no solution possible.\n");
return;
}
// Step 5: Normalize second row
b[1] /= a[1][1];
a[0][1] /= a[1][1]; // Update the pivot column for 'y'
// Step 6: Back-substitution to find y
b[0] -= a[0][1] * b[1];
}
int main() {
float a[2][2] = {{1, 2}, {3, 4}}, b[2] = {5, 6}; // Coefficients and constants
gauss_elimination(a, b);
// The matrix now represents the coefficients for the new variables after elimination
printf("Solution in terms of new variables:\ny = %.2f\n", b[0]);
printf("x = %.2f\n", -b[1]); // We negate the value because we swapped 'x' with its negation earlier
return 0;
}
```
阅读全文
相关推荐
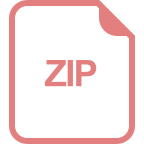







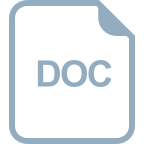






