用c++代码以三元组顺序表的数据类型存储矩阵,设计并实现矩阵的输入、输出和转置算法。
时间: 2024-10-24 13:15:57 浏览: 27
在C++中,我们可以使用三元组(std::tuple)作为数据元素来构建一个动态数组(类似链表),实现矩阵的存储。由于三元组可以包含任意类型的三个元素,我们可以将其用于存储矩阵的行和列坐标以及对应的值。
首先,我们需要定义一个表示矩阵元素的结构体:
```cpp
#include <iostream>
#include <tuple>
// 定义矩阵元素(i, j, value)
using MatrixElement = std::tuple<int, int, double>;
```
然后创建一个动态数组(链表)来存储矩阵:
```cpp
class DynamicMatrix {
private:
std::list<MatrixElement> elements;
int rows, cols;
public:
// 构造函数,初始化矩阵大小
DynamicMatrix(int r, int c) : rows(r), cols(c) {}
// 输入矩阵
void inputMatrix(const std::vector<std::vector<double>>& matrix) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
elements.push_back(std::make_tuple(i, j, matrix[i][j]));
}
}
}
// 输出矩阵
void outputMatrix() const {
for (const auto& element : elements) {
int row, col;
double val;
std::tie(row, col, val) = element;
std::cout << "[ " << row << ", " << col << " ] = " << val << " ";
}
std::cout << "\n";
}
// 转置矩阵
void transpose() {
DynamicMatrix transposed(cols, rows);
for (const auto& element : elements) {
int oldRow, oldCol, val;
std::tie(oldRow, oldCol, val) = element;
transposed.elements.push_back(std::make_tuple(oldCol, oldRow, val));
}
elements.swap(transposed.elements);
}
};
```
现在你可以使用这个`DynamicMatrix`类来创建、输入、输出和转置矩阵了:
```cpp
int main() {
DynamicMatrix mat(3, 3); // 创建一个3x3矩阵
std::vector<std::vector<double>> inputMatrix {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
mat.inputMatrix(inputMatrix);
mat.outputMatrix(); // 输出原始矩阵
mat.transpose();
mat.outputMatrix(); // 输出转置后的矩阵
return 0;
}
```
阅读全文
相关推荐
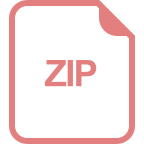
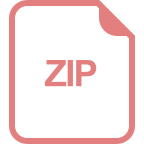
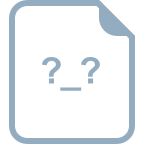






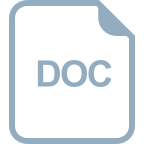
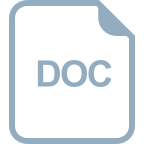
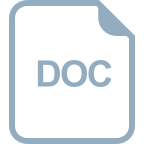
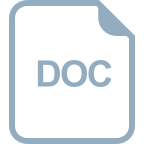
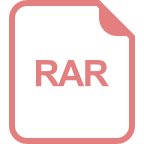
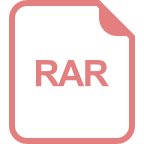
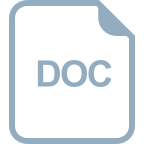
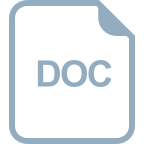
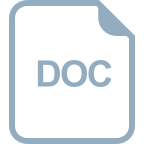
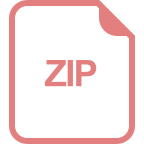