剪枝函数分支限界法0-1背包问题c语言
时间: 2023-12-28 14:01:42 浏览: 303
剪枝函数分支限界法是一种常用于解决0-1背包问题的算法。在C语言中,我们可以使用递归的方式实现这一算法。
首先,我们需要定义一个结构体来表示物品的信息,包括物品的重量和价值。然后,我们可以定义一个递归函数来不断地搜索可能的解,并在搜索过程中使用剪枝函数来减少搜索空间。
在递归函数中,我们可以通过参数来表示当前的搜索状态,包括当前已经选择的物品、剩余的空间和当前已经获得的价值。然后在每一步中,我们可以依次尝试选择或不选择当前物品,然后继续搜索下一个物品的情况,直到搜索完所有的物品为止。
在搜索的过程中,我们可以使用剪枝函数来判断当前的搜索状态是否有可能获得比当前最优解更好的结果。如果当前状态不可能获得更好的解,我们就可以剪掉当前分支,从而减少不必要的搜索。
最后,当搜索完所有的可能情况后,我们就可以得到问题的最优解。这种方法能够在搜索空间较大时显著减少搜索时间,提高算法效率。
在C语言中实现剪枝函数分支限界法的0-1背包问题,需要谨慎处理递归和剪枝函数的逻辑,同时需要合理地定义数据结构和参数传递方式,以确保算法的正确性和高效性。同时,需要对搜索状态和剪枝条件有一个清晰的认识,以找到合适的剪枝点,从而减少搜索空间。
相关问题
分支限界法解决01背包问题c语言实现
好的,这里提供一个基于C语言的分支限界法解决01背包问题的实现。下面的代码实现了一个求解01背包问题的函数,函数的参数包括物品数目n、背包容量c、每个物品的重量w和价值v,以及函数返回的最大价值。代码中使用了一个结构体Item来表示每个物品,其中包含了物品的重量和价值。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int weight;
int value;
} Item;
// 比较函数,按照每个物品的单位价值从大到小排序
int cmp(const void* a, const void* b) {
Item* itemA = (Item*)a;
Item* itemB = (Item*)b;
double uA = (double)itemA->value / itemA->weight;
double uB = (double)itemB->value / itemB->weight;
if (uA > uB) {
return -1;
} else if (uA < uB) {
return 1;
} else {
return 0;
}
}
// 分支限界法求解01背包问题
double knapsack(int n, int c, int* w, int* v) {
Item* items = (Item*)malloc(n * sizeof(Item));
for (int i = 0; i < n; i++) {
items[i].weight = w[i];
items[i].value = v[i];
}
qsort(items, n, sizeof(Item), cmp);
double maxV = 0.0;
int curW = 0;
int curV = 0;
int* mark = (int*)calloc(n, sizeof(int));
int* curMark = (int*)calloc(n, sizeof(int));
double bound = 0.0;
int k = 0;
while (1) {
if (curW + items[k].weight <= c) {
curW += items[k].weight;
curV += items[k].value;
curMark[k] = 1;
} else {
curMark[k] = (c - curW) * 1.0 / items[k].weight;
curW = c;
curV += curMark[k] * items[k].value;
}
if (curV > maxV) {
maxV = curV;
for (int i = 0; i < n; i++) {
mark[i] = curMark[i];
}
}
if (k == n - 1) {
break;
}
bound = curV + (c - curW) * (double)items[k + 1].value / items[k + 1].weight;
if (bound <= maxV) {
break;
}
k++;
}
free(curMark);
free(mark);
free(items);
return maxV;
}
int main() {
int n = 4;
int c = 15;
int w[] = {2, 3, 5, 7};
int v[] = {10, 5, 15, 7};
double maxV = knapsack(n, c, w, v);
printf("Max value: %.1f\n", maxV);
return 0;
}
```
在这个实现中,我们首先将所有物品按照单位价值从大到小排序,然后按照顺序依次选择物品,直到背包装满或者没有物品可选。在每一次选择物品的过程中,我们计算剩余物品的价值上限,如果这个上限已经小于当前最优解,就可以剪枝,不再扩展该节点。如果这个上限大于当前最优解,则继续扩展该节点,直到找到一个完整的解或者搜索完整棵树。
希望这个C语言实现的例子能够对您有所帮助。
优先队列分支限界法实现0/1背包C语言
以下是使用优先队列分支限界法实现0/1背包的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 物品结构体
typedef struct item {
int weight; // 物品重量
int value; // 物品价值
double density; // 物品密度,即单位重量的价值
} Item;
// 优先队列结构体
typedef struct queue {
int level; // 节点所处的层数
int profit; // 当前节点的价值
int weight; // 当前节点的重量
double bound; // 当前节点的价值上界
} Queue;
// 构造优先队列节点
Queue* newQueue(int level, int profit, int weight, double bound) {
Queue* q = (Queue*)malloc(sizeof(Queue));
q->level = level;
q->profit = profit;
q->weight = weight;
q->bound = bound;
return q;
}
// 比较函数,用于优先队列排序
int cmp(const void* a, const void* b) {
Queue* q1 = *(Queue**)a;
Queue* q2 = *(Queue**)b;
return q2->bound - q1->bound;
}
// 优先队列分支限界法求解0/1背包问题
int knapsack(Item* items, int n, int capacity) {
// 按照物品密度从大到小排序
for (int i = 0; i < n; i++) {
items[i].density = (double)items[i].value / (double)items[i].weight;
}
qsort(items, n, sizeof(Item), cmp);
// 初始化优先队列,将根节点加入队列中
Queue** queue = (Queue**)malloc(sizeof(Queue*) * (n + 1));
Queue* root = newQueue(-1, 0, 0, 0.0);
queue[0] = root;
int front = 0, rear = 1;
int maxProfit = 0;
// 迭代搜索优先队列中的节点
while (front < rear) {
// 取出队首节点
Queue* q = queue[front++];
if (q->bound > maxProfit) { // 可行性剪枝
if (q->level == n - 1) { // 到达叶子节点,更新最大价值
maxProfit = q->profit;
} else {
// 产生左儿子节点(装入该物品)
int leftLevel = q->level + 1;
int leftProfit = q->profit + items[leftLevel].value;
int leftWeight = q->weight + items[leftLevel].weight;
double leftBound = q->bound;
if (leftWeight <= capacity) { // 可行性剪枝
leftBound += (double)items[leftLevel + 1].value / (double)items[leftLevel + 1].weight * (capacity - leftWeight);
Queue* left = newQueue(leftLevel, leftProfit, leftWeight, leftBound);
queue[rear++] = left;
}
// 产生右儿子节点(不装入该物品)
int rightLevel = q->level + 1;
int rightProfit = q->profit;
int rightWeight = q->weight;
double rightBound = q->bound;
if (rightLevel < n - 1) { // 限界剪枝
rightBound += (double)items[rightLevel + 1].value / (double)items[rightLevel + 1].weight * (capacity - rightWeight);
Queue* right = newQueue(rightLevel, rightProfit, rightWeight, rightBound);
queue[rear++] = right;
}
}
}
}
// 释放内存
for (int i = 0; i < rear; i++) {
free(queue[i]);
}
free(queue);
return maxProfit;
}
int main() {
Item items[] = {{2, 12}, {1, 10}, {3, 20}, {2, 15}};
int n = sizeof(items) / sizeof(items[0]);
int capacity = 5;
int maxProfit = knapsack(items, n, capacity);
printf("Max profit: %d\n", maxProfit);
return 0;
}
```
代码中,先将物品按照密度从大到小排序,然后构造根节点,并将其加入优先队列中。接着,迭代搜索队列中的节点,对于每个节点,产生左右两个儿子节点,并根据可行性剪枝和限界剪枝来确定是否将其加入队列中。最终,搜索到叶子节点或者队列为空时,返回最大价值。
以上就是使用优先队列分支限界法实现0/1背包问题的C语言代码。
阅读全文
相关推荐
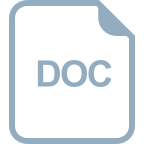
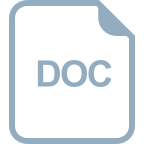
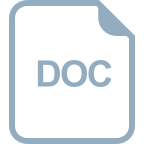
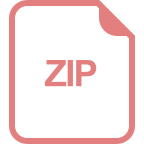
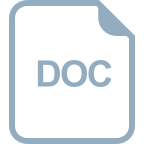
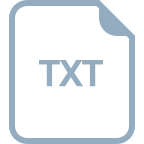
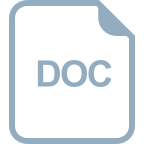
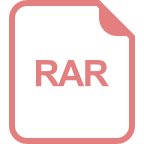
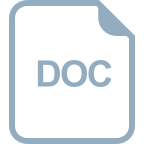
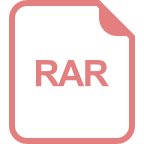
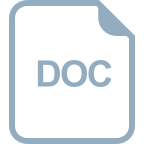




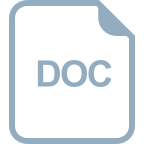
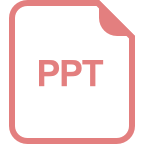