_, threshold = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
时间: 2023-11-07 17:54:48 浏览: 180
根据提供的代码,`_, threshold = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)`是一个二值化图像的阈值处理方法。在这个方法中,使用了灰度图像`gray`,将像素值大于或等于127的像素设置为255(白色),像素值小于127的像素设置为0(黑色)。阈值处理后的结果存储在`threshold`变量中。
相关问题
threshold = 30 _,thresh = cv2.threshold(diff,threshold,255,cv2.THRESH_BINARY)代码的含义以及源代码讲解
这段代码的含义是通过 OpenCV 库中的 `threshold` 函数来对输入图像 `diff` 进行二值化处理,将小于阈值 `threshold` 的像素点赋值为 0,大于等于阈值的像素点赋值为 255。
具体来说,`cv2.threshold` 函数的参数含义如下:
- `diff`:输入图像,可以是灰度图或者彩色图像;
- `threshold`:阈值,如代码中的 30;
- `255`:当像素值大于等于阈值时,所赋的像素值;
- `cv2.THRESH_BINARY`:二值化类型,这里是二值化阈值化类型,即将小于阈值的像素点赋值为 0,大于等于阈值的像素点赋值为 255。
源代码讲解:
```python
threshold = 30
_, thresh = cv2.threshold(diff, threshold, 255, cv2.THRESH_BINARY)
```
首先定义了阈值 `threshold` 为 30,然后调用 `cv2.threshold` 函数将 `diff` 二值化处理,并将结果保存在 `thresh` 变量中。由于在这里并不需要返回阈值,因此使用了 `_` 占位符来代替。最后,`thresh` 变量即为二值化后的图像。
报错如何解决,Exception in Tkinter callback Traceback (most recent call last): File "C:\Users\86135\AppData\Local\Programs\Python\Python39\lib\tkinter\__init__.py", line 1892, in __call__ return self.func(*args) File "F:\pycharm\实验5\Demo5_2.py", line 38, in count_cells _, contours, _ = cv2.findContours(self.thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) ValueError: not enough values to unpack (expected 3, got 2)
这个错误是因为cv2库在新的版本中,`cv2.findContours()`函数返回值的格式发生了改变。在旧版本中,返回值是3个,包括轮廓、层次结构和近似轮廓点。而在新版本中,返回值是2个,只包括轮廓和层次结构。
要解决这个错误,可以将代码中的3个变量都替换为2个变量,或者使用新版本cv2库的返回值。以下是两种方法的代码示例:
方法一:将3个变量都替换为2个变量
```python
import cv2
import numpy as np
import tkinter as tk
from tkinter import filedialog
class CellCounter:
def __init__(self):
self.root = tk.Tk()
self.root.title("Cell Counter")
self.root.geometry("400x300")
self.img = None
self.thresh = None
self.count = 0
self.img_label = tk.Label(self.root, text="No image selected")
self.img_label.pack(pady=10)
self.btn_select = tk.Button(self.root, text="Select Image", command=self.select_image)
self.btn_select.pack(pady=10)
self.btn_count = tk.Button(self.root, text="Count Cells", command=self.count_cells)
self.btn_count.pack(pady=10)
self.count_label = tk.Label(self.root, text="Cell Count: 0")
self.count_label.pack(pady=10)
def select_image(self):
path = filedialog.askopenfilename(filetypes=[("Image Files", "*.jpg;*.jpeg;*.png;*.bmp")])
if path:
self.img = cv2.imread(path)
self.img_label.config(text="Image: {}".format(path))
self.thresh = None
self.count = 0
self.count_label.config(text="Cell Count: 0")
def count_cells(self):
if self.img is None:
return
if self.thresh is None:
gray = cv2.cvtColor(self.img, cv2.COLOR_BGR2GRAY)
_, self.thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
contours, _ = cv2.findContours(self.thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
self.count = len(contours)
self.count_label.config(text="Cell Count: {}".format(self.count))
def run(self):
self.root.mainloop()
if __name__ == "__main__":
app = CellCounter()
app.run()
```
方法二:使用新版本cv2库的返回值
```python
import cv2
import numpy as np
import tkinter as tk
from tkinter import filedialog
class CellCounter:
def __init__(self):
self.root = tk.Tk()
self.root.title("Cell Counter")
self.root.geometry("400x300")
self.img = None
self.thresh = None
self.count = 0
self.img_label = tk.Label(self.root, text="No image selected")
self.img_label.pack(pady=10)
self.btn_select = tk.Button(self.root, text="Select Image", command=self.select_image)
self.btn_select.pack(pady=10)
self.btn_count = tk.Button(self.root, text="Count Cells", command=self.count_cells)
self.btn_count.pack(pady=10)
self.count_label = tk.Label(self.root, text="Cell Count: 0")
self.count_label.pack(pady=10)
def select_image(self):
path = filedialog.askopenfilename(filetypes=[("Image Files", "*.jpg;*.jpeg;*.png;*.bmp")])
if path:
self.img = cv2.imread(path)
self.img_label.config(text="Image: {}".format(path))
self.thresh = None
self.count = 0
self.count_label.config(text="Cell Count: 0")
def count_cells(self):
if self.img is None:
return
if self.thresh is None:
gray = cv2.cvtColor(self.img, cv2.COLOR_BGR2GRAY)
_, self.thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
_, contours = cv2.findContours(self.thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
self.count = len(contours)
self.count_label.config(text="Cell Count: {}".format(self.count))
def run(self):
self.root.mainloop()
if __name__ == "__main__":
app = CellCounter()
app.run()
```
希望这些方法可以帮助你解决报错问题!
阅读全文
相关推荐
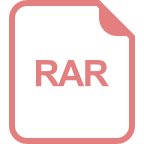
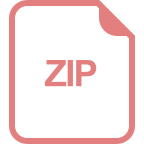
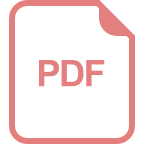













