from tensorflow.keras.datasets import mnist (x_train, y_train), (x_test, y_test) = mnist.load_data() print("Training image shape:", x_train.shape) # (60000, 28, 28) print("Training label shape:", y_train.shape) # (60000,) print("First five training labels:", y_train[:5]) # array([5 0 4 1 9], dtype=uint8) # ### IO API for image classification # # In[ ]: import autokeras as ak # Initialize the IO API. io_model = ak.AutoModel( inputs=ak.ImageInput(), outputs=ak.ClassificationHead( loss="categorical_crossentropy", metrics=["accuracy"] ), objective="val_loss", tuner="random", max_trials=3, overwrite=True,) # Fit the model with prepared data. # Use the first 100 training samples for 1 epoch as a quick demo. # You may run with the full dataset with 10 epochs, but expect a longer training time. io_model.fit(x_train[:100], y_train[:100], epochs=1,verbose=2) UnicodeDecodeError: 'utf-8' codec can't decode byte 0xd5 in position 200: invalid continuation byte,如何解决,给出具体代码
时间: 2024-04-11 17:30:29 浏览: 141
这个错误是由于代码中的某个地方出现了一个无效的续字符,导致无法解码为utf-8格式。具体来说,"UnicodeDecodeError: 'utf-8' codec can't decode byte 0xd5 in position 200: invalid continuation byte" 意味着在位置200出现了一个无效的续字符。
然而,根据你提供的代码,这个错误与你的代码似乎没有直接关联。这是因为你的代码中没有涉及到字符解码操作。因此,这个错误可能是由于其他部分的代码引起的。
要解决这个问题,你可以尝试以下几种方法:
1. 检查你的代码中是否有其他地方使用了字符解码操作,例如读取文件或处理网络请求等。确保在这些地方使用正确的编码格式进行解码操作。
2. 检查你的输入数据是否包含特殊字符或不支持的字符。如果有,可以尝试删除或替换这些字符。
3. 确保你的代码中的所有字符串都使用正确的编码格式进行处理。
如果以上方法都无法解决问题,请提供更多关于错误信息和代码的详细信息,以便我能够更好地帮助你解决问题。
相关问题
import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense from tensorflow.keras.layers import LSTM from tensorflow.keras.optimizers import Adam # 载入数据 mnist = tf.keras.datasets.mnist (x_train, y_train), (x_test, y_test) = mnist.load_data() x_train, x_test = x_train / 255.0, x_test / 255.0 print(x_train.shape, y_train.shape) # 创建模型 # y_train = tf.keras.utils.to_categorical(y_train,num_classes=10) # y_test = tf.keras.utils.to_categorical(y_test,num_classes=10) # 数据长度-一行有28个像素 input_size = 28 # 序列长度-一共有28行 time_steps = 28 # 隐藏层cell个数 cell_size = 50 # 创建模型 model = Sequential() # 循环神经网络 model.add(LSTM( units = cell_size, # 输出 input_shape = (time_steps,input_size), #输入 )) # 输出层 model.add(Dense(10,activation='softmax')) # 定义优化器 adam = Adam(lr=1e-3) # 定义优化器,loss function,训练过程中计算准确率 model.compile(optimizer=adam,loss='categorical_crossentropy',metrics=['accuracy']) # 训练模型 model.fit(x_train,y_train,batch_size=64,epochs=10) # 评估模型 loss,accuracy = model.evaluate(x_test,y_test) print('test loss',loss) print('test accuracy',accuracy)
这是一个使用Keras框架搭建的LSTM模型,用于对MNIST数据集进行分类。主要的步骤包括:
1. 载入数据集
2. 创建模型,其中包括一个LSTM层和一个输出层
3. 定义优化器、损失函数和评估指标
4. 训练模型
5. 评估模型
具体来说,这个模型使用了一个LSTM层,用于处理序列数据。输入数据是28行28列的图像数据,LSTM层的输入形状为(28, 28)。隐层单元数为50,输出层使用softmax激活函数输出10个类别的概率。
在模型编译时,使用了Adam优化器和交叉熵损失函数,同时计算了准确率来评估模型性能。
在训练时,采用了64个样本作为一个batch,训练10个epoch。
最后,使用测试集评估模型的性能,输出了测试集上的损失和准确率。
请将此代码修改为tensorflow2.7,cuda11.2版本的代码 import tensorflow as tf from tensorflow import keras from tensorflow.keras.datasets import mnist from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, ReLU from tensorflow.keras.utils import to_categorical # 加载MNIST数据集 (x_train, y_train), (x_test, y_test) = mnist.load_data() # 数据预处理 x_train = x_train.astype("float32") / 255.0 x_test = x_test.astype("float32") / 255.0 x_train = tf.expand_dims(x_train, axis=3) x_test = tf.expand_dims(x_test, axis=3) y_train = to_categorical(y_train, num_classes=10) y_test = to_categorical(y_test, num_classes=10) # 构建LeNet-5模型 model = Sequential([ Conv2D(6, kernel_size=3, strides=1), MaxPooling2D(pool_size=2, strides=2), ReLU(), Conv2D(16, kernel_size=3, strides=1), MaxPooling2D(pool_size=2, strides=2), ReLU(), Flatten(), Dense(units=120, activation='relu'), Dense(units=84, activation='relu'), Dense(units=10, activation='softmax') ]) # 编译模型 model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy']) # 训练模型 model.fit(x_train, y_train, batch_size=128, epochs=30, validation_data=(x_test, y_test)) # 在测试集上输出精度 test_loss, test_acc = model.evaluate(x_test, y_test) print('Test accuracy:', test_acc)
这是一个类似的 TensorFlow 2.7 和 CUDA 11.2 版本的代码示例:
```python
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, ReLU
from tensorflow.keras.utils import to_categorical
# Set GPU device
gpus = tf.config.experimental.list_physical_devices('GPU')
if gpus:
try:
tf.config.experimental.set_visible_devices(gpus[0], 'GPU')
tf.config.experimental.set_memory_growth(gpus[0], True)
print("Using GPU:", tf.test.gpu_device_name())
except RuntimeError as e:
print(e)
# Load MNIST dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Preprocess data
x_train = x_train.astype("float32") / 255.0
x_test = x_test.astype("float32") / 255.0
x_train = tf.expand_dims(x_train, axis=3)
x_test = tf.expand_dims(x_test, axis=3)
y_train = to_categorical(y_train, num_classes=10)
y_test = to_categorical(y_test, num_classes=10)
# Define LeNet-5 model
model = Sequential([
Conv2D(6, kernel_size=3, strides=1),
MaxPooling2D(pool_size=2, strides=2),
ReLU(),
Conv2D(16, kernel_size=3, strides=1),
MaxPooling2D(pool_size=2, strides=2),
ReLU(),
Flatten(),
Dense(units=120, activation='relu'),
Dense(units=84, activation='relu'),
Dense(units=10, activation='softmax')
])
# Compile model
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# Train model
model.fit(x_train, y_train, batch_size=128, epochs=30, validation_data=(x_test, y_test))
# Evaluate model on test set
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
这个代码实现了一个 LeNet-5 模型,用于识别 MNIST 手写数字。它使用了 TensorFlow 2.7 和 CUDA 11.2 版本,并且在 GPU 上训练模型。在代码中,我们首先检查是否有可用的 GPU 设备,并设置 TensorFlow 只使用第一个 GPU 设备。然后,我们加载 MNIST 数据集并对其进行预处理。接下来,我们定义了一个包含两个卷积层和三个全连接层的 LeNet-5 模型,并使用 Adam 优化器和交叉熵损失函数编译了模型。最后,我们在训练集上训练模型,并在测试集上评估模型的准确性。
阅读全文
相关推荐
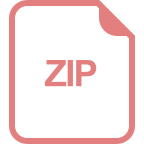
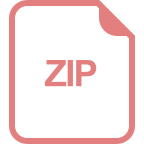
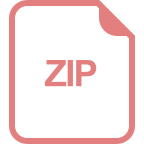













