stm32红外循迹小车的代码
时间: 2024-05-14 08:10:07 浏览: 130
当涉及到STM32红外循迹小车的代码时,首先需要明确你使用的是哪个型号的STM32开发板以及你所使用的红外传感器。不同的开发板和传感器可能会有不同的接口和驱动程序。
一般来说,STM32红外循迹小车的代码可以分为以下几个部分:
1. 初始化:包括初始化GPIO引脚、串口、定时器等外设,并设置红外传感器的引脚为输入模式。
2. 红外传感器读取:通过读取红外传感器的引脚状态,获取红外传感器的数据。根据具体的传感器型号,可能需要进行模拟输入的读取或者数字输入的读取。
3. 控制算法:根据红外传感器读取到的数据,进行控制算法的设计。常见的算法包括PID控制、模糊控制等。根据传感器读取到的数据,判断小车应该向前、向后、左转还是右转,并控制电机的转动。
4. 电机控制:根据控制算法的结果,控制电机的转动。可以通过PWM信号控制电机的速度和方向。
下面是一个简单的示例代码,用于演示红外循迹小车的基本功能:
```cpp
#include "stm32f4xx.h"
// 定义红外传感器引脚
#define IR_SENSOR_PIN GPIO_Pin_0
#define IR_SENSOR_GPIO GPIOA
// 定义电机引脚
#define MOTOR_PIN1 GPIO_Pin_1
#define MOTOR_PIN2 GPIO_Pin_2
#define MOTOR_GPIO GPIOB
// 初始化函数
void init() {
// 初始化GPIO引脚
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA | RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = IR_SENSOR_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(IR_SENSOR_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = MOTOR_PIN1 | MOTOR_PIN2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(MOTOR_GPIO, &GPIO_InitStructure);
}
// 读取红外传感器数据
int readIRSensor() {
return GPIO_ReadInputDataBit(IR_SENSOR_GPIO, IR_SENSOR_PIN);
}
// 控制电机转动
void controlMotor(int direction) {
if (direction == 0) {
// 停止
GPIO_ResetBits(MOTOR_GPIO, MOTOR_PIN1);
GPIO_ResetBits(MOTOR_GPIO, MOTOR_PIN2);
} else if (direction == 1) {
// 向前
GPIO_SetBits(MOTOR_GPIO, MOTOR_PIN1);
GPIO_ResetBits(MOTOR_GPIO, MOTOR_PIN2);
} else if (direction == 2) {
// 向后
GPIO_ResetBits(MOTOR_GPIO, MOTOR_PIN1);
GPIO_SetBits(MOTOR_GPIO, MOTOR_PIN2);
} else if (direction == 3) {
// 左转
GPIO_ResetBits(MOTOR_GPIO, MOTOR_PIN1);
GPIO_ResetBits(MOTOR_GPIO, MOTOR_PIN2);
} else if (direction == 4) {
// 右转
GPIO_SetBits(MOTOR_GPIO, MOTOR_PIN1);
GPIO_SetBits(MOTOR_GPIO, MOTOR_PIN2);
}
}
int main() {
init();
while (1) {
int sensorData = readIRSensor();
// 根据传感器数据进行控制算法
int direction = 0;
if (sensorData == 0b00001) {
direction = 1; // 向前
} else if (sensorData == 0b00010) {
direction = 4; // 右转
} else if (sensorData == 0b00100) {
direction = 3; // 左转
} else if (sensorData == 0b01000) {
direction = 2; // 向后
} else {
direction = 0; // 停止
}
controlMotor(direction);
}
}
```
请注意,以上代码仅为示例,具体的代码实现可能会因为使用的开发板和传感器不同而有所差异。你需要根据你所使用的硬件进行相应的修改和适配。
阅读全文
相关推荐
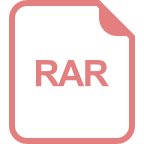
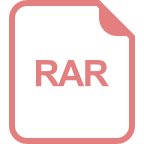
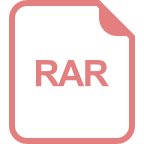
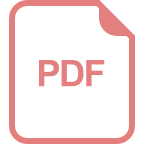
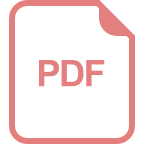
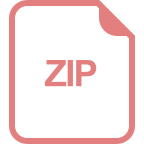