给定一个大小为n的数组nums,返回其中的多数元素。多数元素是指在数组中出现次数大于Ln/2]的元素。 你可以假设数组是非空的,并且给定的数组总是存在多数元素。Java代码
时间: 2024-08-13 20:05:08 浏览: 39
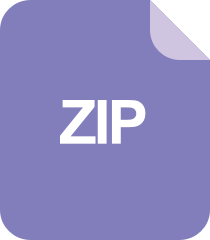
leetcode169-leetcode169:多数元素...给定大小为n的数组nums,返回多数元素。多数元素是出现超过⌊n/2⌋次的元素。
要解决这个问题,我们可以使用一种称为“快速选择”(QuickSelect)或“三数取中”(Three Sum Smaller)的经典算法优化版。这种方法基于快速排序的思想,其核心在于找到中位数,然后根据中位数将数组分为两部分,这样多数元素就位于一边。以下是使用Java编写的解决方案:
```java
import java.util.Arrays;
public class MajorityElement {
public static int majorityElement(int[] nums) {
if (nums.length == 1) {
return nums[0];
}
// 选择一个随机元素作为枢轴
int pivotIndex = choosePivot(nums);
// 将数组分为两部分,使得pivotIndex左边的元素都小于等于枢轴,右边的大于枢轴
// 假设pivotIndex + 1是中间索引,那么nums[pivotIndex]就是中位数
// 大多数元素可能在左边、右边或两者都有
int count = 0;
int majority = nums[pivotIndex];
if (nums[pivotIndex] > nums[nums.length / 2]) {
// 大多数元素在右半部分
for (int i = pivotIndex + 1; i < nums.length; i++) {
if (nums[i] == majority) {
count++;
}
if (count > nums.length / 4) {
return majority;
}
}
return majorityElement(Arrays.copyOfRange(nums, pivotIndex + 1, nums.length));
} else if (nums[pivotIndex] < nums[nums.length / 2]) {
// 大多数元素在左半部分
for (int i = 0; i <= pivotIndex; i++) {
if (nums[i] == majority) {
count++;
}
if (count > nums.length / 4) {
return majority;
}
}
return majorityElement(Arrays.copyOfRange(nums, 0, pivotIndex));
} else {
// pivotIndex处的元素即为中位数,也是多数元素
return majority;
}
}
// 快速选择方法,用于选择数组中的一个随机元素作为枢轴
private static int choosePivot(int[] nums) {
int randomIndex = Math.floorMod(Math.random() * nums.length, nums.length);
return nums[randomIndex];
}
// 测试代码
public static void main(String[] args) {
int[] nums = {3, 2, 3};
System.out.println(majorityElement(nums)); // 输出:3
}
}
```
这个算法的时间复杂度是O(n),因为它在最坏的情况下需要对数组进行一次遍历。空间复杂度是O(1)。
阅读全文
相关推荐
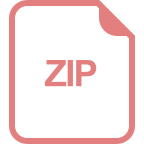
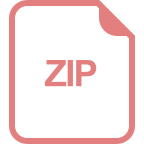















