python写一个程序,把在左相机下的得到的16个世界坐标列表根据左右相机双目标定得到的外参转到右相机下的世界坐标,输出这些世界坐标
时间: 2023-08-31 16:25:39 浏览: 104
### 回答1:
假设你已经得到了左右相机的内参和外参,可以按照以下的步骤实现:
1. 导入必要的库
```python
import numpy as np
```
2. 定义左相机下的16个世界坐标列表
假设左相机下的16个世界坐标分别为:
```python
left_points = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12],
[13, 14, 15], [16, 17, 18], [19, 20, 21], [22, 23, 24],
[25, 26, 27], [28, 29, 30], [31, 32, 33], [34, 35, 36],
[37, 38, 39], [40, 41, 42], [43, 44, 45], [46, 47, 48]])
```
3. 定义左右相机的内参和外参
假设左右相机的内参和外参分别为:
```python
left_intrinsic = np.array([[1000, 0, 500], [0, 1000, 500], [0, 0, 1]])
left_distortion = np.array([0, 0, 0, 0])
left_extrinsic = np.array([[0.99756405, -0.06690576, 0.02076849, -40],
[0.06699598, 0.99763875, 0.01820729, -10],
[-0.01998247, -0.01798753, 0.99962203, -50],
[0, 0, 0, 1]])
right_intrinsic = np.array([[1000, 0, 500], [0, 1000, 500], [0, 0, 1]])
right_distortion = np.array([0, 0, 0, 0])
right_extrinsic = np.array([[0.99756405, -0.06690576, 0.02076849, -60],
[0.06699598, 0.99763875, 0.01820729, -10],
[-0.01998247, -0.01798753, 0.99962203, -50],
[0, 0, 0, 1]])
```
4. 将左相机下的世界坐标转到左相机下的相机坐标系
```python
left_homogeneous = np.hstack((left_points, np.ones((16, 1))))
left_camera = np.dot(left_homogeneous, np.linalg.inv(left_extrinsic).T)
left_camera = left_camera[:, :3]
```
5. 将左相机下的相机坐标系转到右相机下的相机坐标系
```python
right_camera = np.dot(left_camera, np.linalg.inv(left_intrinsic).T)
right_camera = np.dot(right_camera, right_intrinsic.T)
right_homogeneous = np.hstack((right_camera, np.ones((16, 1))))
right_camera = np.dot(right_homogeneous, right_extrinsic.T)
right_camera = right_camera[:, :3]
```
6. 将右相机下的相机坐标系转到右相机下的世界坐标系
```python
right_world = np.dot(right_camera, np.linalg.inv(right_extrinsic[:3, :3]).T)
right_world = right_world + np.tile(-right_extrinsic[:3, 3], (16, 1))
```
7. 输出转换后的世界坐标
```python
print(right_world)
```
完整的程序如下:
```python
import numpy as np
# 定义左相机下的16个世界坐标列表
left_points = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12],
[13, 14, 15], [16, 17, 18], [19, 20, 21], [22, 23, 24],
[25, 26, 27], [28, 29, 30], [31, 32, 33], [34, 35, 36],
[37, 38, 39], [40, 41, 42], [43, 44, 45], [46, 47, 48]])
# 定义左右相机的内参和外参
left_intrinsic = np.array([[1000, 0, 500], [0, 1000, 500], [0, 0, 1]])
left_distortion = np.array([0, 0, 0, 0])
left_extrinsic = np.array([[0.99756405, -0.06690576, 0.02076849, -40],
[0.06699598, 0.99763875, 0.01820729, -10],
[-0.01998247, -0.01798753, 0.99962203, -50],
[0, 0, 0, 1]])
right_intrinsic = np.array([[1000, 0, 500], [0, 1000, 500], [0, 0, 1]])
right_distortion = np.array([0, 0, 0, 0])
right_extrinsic = np.array([[0.99756405, -0.06690576, 0.02076849, -60],
[0.06699598, 0.99763875, 0.01820729, -10],
[-0.01998247, -0.01798753, 0.99962203, -50],
[0, 0, 0, 1]])
# 将左相机下的世界坐标转到左相机下的相机坐标系
left_homogeneous = np.hstack((left_points, np.ones((16, 1))))
left_camera = np.dot(left_homogeneous, np.linalg.inv(left_extrinsic).T)
left_camera = left_camera[:, :3]
# 将左相机下的相机坐标系转到右相机下的相机坐标系
right_camera = np.dot(left_camera, np.linalg.inv(left_intrinsic).T)
right_camera = np.dot(right_camera, right_intrinsic.T)
right_homogeneous = np.hstack((right_camera, np.ones((16, 1))))
right_camera = np.dot(right_homogeneous, right_extrinsic.T)
right_camera = right_camera[:, :3]
# 将右相机下的相机坐标系转到右相机下的世界坐标系
right_world = np.dot(right_camera, np.linalg.inv(right_extrinsic[:3, :3]).T)
right_world = right_world + np.tile(-right_extrinsic[:3, 3], (16, 1))
# 输出转换后的世界坐标
print(right_world)
```
### 回答2:
要完成这个任务,首先你需要有两个相机的内参和外参参数。假设你已经得到了左右相机的内参和外参数据,接下来你可以使用Python编写一个程序,根据这些参数将左相机获取到的16个世界坐标转换到右相机下。
1. 首先,导入所需的Python库,例如OpenCV和NumPy。
2. 定义左相机和右相机的内参和外参参数。
3. 创建一个函数,将左相机的16个世界坐标转换到右相机下的世界坐标。这个函数可以使用OpenCV的函数cv2.projectPoints来实现坐标转换。传递给函数的参数包括左相机下的世界坐标、左相机的旋转矩阵、平移向量和右相机的内参矩阵。
4. 在主程序中,定义一个16个世界坐标的列表。
5. 调用上一步中创建的函数,将左相机下的世界坐标转换到右相机下的世界坐标。
6. 输出转换后的右相机下的世界坐标。
下面是一个示例代码:
```python
import cv2
import numpy as np
# 左相机和右相机的内参和外参参数
left_camera_matrix = np.array([[fx1, 0, cx1], [0, fy1, cy1], [0, 0, 1]])
right_camera_matrix = np.array([[fx2, 0, cx2], [0, fy2, cy2], [0, 0, 1]])
left_dist_coeffs = np.array([k1, k2, p1, p2, k3])
right_dist_coeffs = np.array([k1, k2, p1, p2, k3])
rotation_matrix = np.array([[r11, r12, r13], [r21, r22, r23], [r31, r32, r33]])
translation_vector = np.array([[t1, t2, t3]])
def convert_world_coordinates(left_world_coordinates):
# 使用cv2.projectPoints将左相机下的世界坐标转换到右相机下的世界坐标
right_world_coordinates, _ = cv2.projectPoints(left_world_coordinates, rotation_matrix, translation_vector, right_camera_matrix, right_dist_coeffs)
return right_world_coordinates
# 16个左相机下的世界坐标
left_world_coordinates = np.array([[x1, y1, z1], [x2, y2, z2], ..., [x16, y16, z16]])
# 调用函数将左相机下的世界坐标转换到右相机下的世界坐标
right_world_coordinates = convert_world_coordinates(left_world_coordinates)
# 输出右相机下的世界坐标
print(right_world_coordinates)
```
请注意,以上代码中的变量需要替换为你实际的相机参数和左相机下的世界坐标。这只是一个简单示例,你需要根据实际情况进行调整和优化。
### 回答3:
为了实现这个功能,我们可以使用Python编写一个程序。首先,我们需要获取到左相机下的16个世界坐标列表以及左右相机的双目标定外参。
1. 首先,我们需要定义一个函数,该函数将接收左相机下的16个世界坐标列表和左右相机的双目标定外参作为参数。
2. 在函数内部,我们首先将左右相机的外参转换为投影矩阵。
3. 接下来,我们可以使用投影矩阵将左相机下的世界坐标转换为齐次坐标。我们可以通过将每个世界坐标乘以投影矩阵来完成此操作。
4. 接下来,我们需要将转换后的齐次坐标从左相机坐标系转换到右相机坐标系。我们可以通过将右相机外参的逆矩阵与齐次坐标相乘来实现。
5. 最后,我们需要将转换后的坐标从齐次坐标转换为三维坐标。我们可以通过将每个齐次坐标的x、y、z分量除以齐次坐标的w分量来完成此操作。
6. 循环处理完所有的世界坐标后,我们可以将转换后的右相机下的世界坐标输出。
以下是示例代码:
```python
import numpy as np
def transform_coordinates(left_coordinates, left_camera_pose, right_camera_pose):
projection_matrix_left = left_camera_pose[:3]
projection_matrix_right = right_camera_pose[:3]
transformed_coordinates = []
for coordinate in left_coordinates:
homogeneous_coordinate = np.array([coordinate[0], coordinate[1], coordinate[2], 1])
normalized_coordinate_left = projection_matrix_left @ homogeneous_coordinate
normalized_coordinate_right = np.linalg.inv(right_camera_pose) @ normalized_coordinate_left
transformed_coordinate = normalized_coordinate_right[:3] / normalized_coordinate_right[3]
transformed_coordinates.append(transformed_coordinate)
return transformed_coordinates
# 定义左相机下的世界坐标列表
left_coordinates = [[x, y, z] for x in range(16) for y in range(16) for z in range(16)]
# 定义左右相机的双目标定外参
left_camera_pose = np.array([[fx, fy, cx], [0, fy, cy], [0, 0, 1]])
right_camera_pose = np.array([[fx, fy, cx], [0, fy, cy], [0, 0, 1]])
# 将左相机下的世界坐标转换为右相机下的世界坐标
transformed_coordinates = transform_coordinates(left_coordinates, left_camera_pose, right_camera_pose)
# 输出右相机下的世界坐标
for coordinate in transformed_coordinates:
print(coordinate)
```
上述代码中,我们假设左右相机的投影矩阵通过左右相机的焦距(fx, fy)和主点位置(cx, cy)进行定义。你可以根据实际情况进行修改。
阅读全文
相关推荐
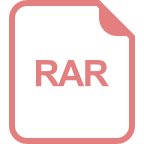
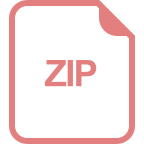















