C++实现:当直线方程为x=1时,求点到该直线的距离
时间: 2024-03-17 10:42:12 浏览: 23
如果直线方程为x=1,那么该直线与y轴平行,其法向量为(1,0)。对于任意一点P(x,y),其到该直线的距离可以通过以下公式计算:
distance = abs((x - 1) * 0 + (y - 0) * 1) / sqrt(0^2 + 1^2)
化简后可得:
distance = abs(x - 1)
因此,要求点到该直线的距离,只需要求出该点的x坐标与1的差的绝对值即可。以下是C++实现代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x, y; // 输入点的坐标
double distance; // 与直线的距离
cout << "请输入点的坐标(x,y):";
cin >> x >> y;
distance = abs(x - 1);
cout << "该点到直线的距离为:" << distance << endl;
return 0;
}
```
相关问题
c++ 3d求点到直线的距离_3D空间点到直线的距离
在三维空间中,点到直线的距离可以通过向量运算来求解。假设有一个点P和一条直线L,我们可以先求出点P到直线L所在平面的垂线L1,然后计算垂线L1的长度,即为点P到直线L的距离。
具体实现可以按照以下步骤进行:
1. 求直线L的方向向量d和一点P0,构造直线L的参数方程P=P0+td,其中t为实数。
2. 求点P在直线L所在平面上的投影点P1。为了求得P1,可以使用向量运算,即将向量P-P0投影到向量d上,然后加上P0即可。
3. 求垂线L1的长度,即为点P到直线L的距离。可以使用向量运算,即将向量P-P1取模即可。
下面是一个求解点到直线距离的C++函数实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
// 三维点的类定义
class Point3D
{
public:
double x, y, z;
Point3D(double a = 0, double b = 0, double c = 0) : x(a), y(b), z(c) {}
};
// 三维向量的类定义
class Vector3D
{
public:
double x, y, z;
Vector3D(double a = 0, double b = 0, double c = 0) : x(a), y(b), z(c) {}
// 向量点乘
double dot(const Vector3D& v) const
{
return x * v.x + y * v.y + z * v.z;
}
// 向量叉乘
Vector3D cross(const Vector3D& v) const
{
return Vector3D(y * v.z - z * v.y, z * v.x - x * v.z, x * v.y - y * v.x);
}
// 向量取模
double norm() const
{
return sqrt(x * x + y * y + z * z);
}
// 向量归一化
Vector3D normalize() const
{
double n = norm();
return Vector3D(x / n, y / n, z / n);
}
};
// 点到直线的距离
double distance(const Point3D& p, const Point3D& p0, const Vector3D& d)
{
Vector3D v(p.x - p0.x, p.y - p0.y, p.z - p0.z); // 求向量P-P0
Vector3D v1 = v - d.normalize() * d.dot(v) / d.norm(); // 求向量P1-P0
return v1.norm(); // 求垂线长度
}
int main()
{
Point3D p(1, 2, 3);
Point3D p0(0, 0, 0);
Vector3D d(1, 1, 1);
double dist = distance(p, p0, d);
cout << "Distance: " << dist << endl;
return 0;
}
```
上述代码中,Point3D类和Vector3D类分别表示三维点和向量,其中Vector3D类中包含了向量点乘、叉乘、取模和归一化等常用的向量运算。distance函数用于计算点到直线的距离,其中p表示点P,p0表示直线L上的一点P0,d表示直线L的方向向量。函数首先求出向量P-P0,然后求出向量P1-P0,最后求出垂线L1的长度。
c++求点到平面的距离
### 回答1:
点到平面的距离是指从点到平面上的最短距离。在三维空间中,可以通过以下公式来求解点P(x,y,z)到平面Ax+By+Cz+D=0的距离:
d = |Ax + By + Cz + D| / √(A² + B² + C²)
其中,“| |”表示绝对值符号,“√”表示平方根符号。
具体来说,首先需要求出平面的法向量N(A,B,C),然后用点P到平面上任意一点Q的向量PQ与法向量N的点积作为分子,再求出N的模长作为分母即可。
需要注意的是,如果点P在平面上,则点到平面的距离为0;如果法向量N为零向量,则平面不存在,无法计算点到平面的距离。此外,如果平面方程不是标准式,比如写成一般式或点法式,需要先转化为标准式才能应用上述公式。
### 回答2:
点到平面的距离是指从该点向垂直于平面的方向所作的线段的长度,这一长度可以用向量的内积和模长求得。
假设点的坐标为P(x1,y1,z1),平面的方程为Ax+By+Cz+D = 0。首先,将平面的方程变形为法向量N=(A, B, C)和点O(任意一点,假设坐标为(x0,y0,z0))的内积形式,如下所示:
N·(P-O) + D = 0
其中·表示向量的内积。
那么点P到平面的距离h可以表示为:
h = |N·(P-O)| / |N|
其中|N|表示向量N的长度。因此,我们只需要求得向量N的长度和向量N·(P-O)的长度,就可以求得点P到平面的距离h。
具体计算过程如下:
1. 求向量N的长度
|N| = sqrt(A^2 + B^2 + C^2)
2. 求向量N·(P-O)的长度
N·(P-O) = N·P - N·O = Ax1 + By1 + Cz1 - (Ax0 + By0 + Cz0)
3. 由上述公式计算点P到平面的距离h
h = |N·(P-O)| / |N|
需要注意的是,当向量N为单位向量时,上式中的分母|N|可以省略,计算起来会更加简便。
以上就是求点到平面的距离的计算方法。这一方法可以用于各种情况下,比如在三维空间中研究点和平面的关系,求解问题时可以根据具体需要灵活应用。
### 回答3:
首先,我们需要明确点和平面的概念。
点是几何中没有大小、无限小的一个对象,在空间中被表示为一组坐标。
平面是指没有厚度的无限大平面,可以用两个垂直的轴表示,在三维空间中是一个二维的图形。
点和平面之间的距离,指的是点到平面上最近的距离。计算点到平面的距离的方法如下:
假设点的坐标为P(x1,y1,z1),平面的方程为Ax+By+Cz+D=0。
- 求出平面内一点Q的坐标:假设平面上垂直于z轴的直线与该平面的交点为Q,则Q的坐标为(x1,y1,-(A*x1+B*y1+D)/C)。
- 求出向量PQ:向量PQ的坐标为(PQx,PQy,PQz),其中PQx=x1-x2、PQy=y1-y2、PQz=z1-z2。
- 求出平面的法向量:平面的法向量为N(A,B,C)。
- 计算点到平面的距离:点到平面的距离为其在法向量方向上的分量,即点到平面的距离d=|PQ·N|/|N|。
其中,|PQ·N|表示向量PQ与平面法向量N的点积,|N|表示平面法向量N的模长。
举个例子,在三维空间中,点P(2,3,4)到平面2x+3y+4z-5=0的距离该如何求解呢?
首先,按照上述方法,求出平面内一点Q的坐标:Q(x1,y1,z1)= (2,3,(5-2*2-3*3)/4) = (2,3,-1/2)。
然后,求出向量PQ: PQ=(PQx,PQy,PQz)=(2-2,3-3,4-(-1/2))=(0,0,9/2)。
接着,计算平面的法向量N=(2,3,4)。
最后,根据上述公式,求得点P到平面的距离:d=|PQ·N|/|N|=|(0,0,9/2)·(2,3,4)|/sqrt(2^2+3^2+4^2)=9/sqrt(29/2)≈5.212。因此,点P到平面2x+3y+4z-5=0的距离为约5.212。
相关推荐
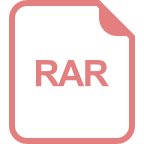
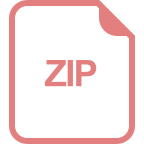












