用ts写一个3个点求顶点法线
时间: 2024-05-09 11:16:33 浏览: 178
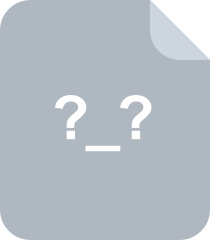
E3D-顶点法线-属性转化.ms
以下是使用 TypeScript 编写的计算三角形顶点法线的代码:
```
interface Vector3 {
x: number;
y: number;
z: number;
}
interface Triangle {
vertices: [Vector3, Vector3, Vector3];
}
function calculateTriangleNormal(triangle: Triangle): Vector3 {
const p1 = triangle.vertices[0];
const p2 = triangle.vertices[1];
const p3 = triangle.vertices[2];
const v1 = { x: p2.x - p1.x, y: p2.y - p1.y, z: p2.z - p1.z };
const v2 = { x: p3.x - p1.x, y: p3.y - p1.y, z: p3.z - p1.z };
const crossProduct = {
x: v1.y * v2.z - v1.z * v2.y,
y: v1.z * v2.x - v1.x * v2.z,
z: v1.x * v2.y - v1.y * v2.x
};
const length = Math.sqrt(
crossProduct.x * crossProduct.x +
crossProduct.y * crossProduct.y +
crossProduct.z * crossProduct.z
);
return {
x: crossProduct.x / length,
y: crossProduct.y / length,
z: crossProduct.z / length
};
}
function calculateVertexNormals(vertices: Vector3[], indices: number[]): Vector3[] {
const vertexNormals: Vector3[] = [];
for (let i = 0; i < vertices.length; i++) {
vertexNormals.push({ x: 0, y: 0, z: 0 });
}
for (let i = 0; i < indices.length; i += 3) {
const triangle: Triangle = {
vertices: [
vertices[indices[i]],
vertices[indices[i + 1]],
vertices[indices[i + 2]]
]
};
const normal = calculateTriangleNormal(triangle);
vertexNormals[indices[i]].x += normal.x;
vertexNormals[indices[i]].y += normal.y;
vertexNormals[indices[i]].z += normal.z;
vertexNormals[indices[i + 1]].x += normal.x;
vertexNormals[indices[i + 1]].y += normal.y;
vertexNormals[indices[i + 1]].z += normal.z;
vertexNormals[indices[i + 2]].x += normal.x;
vertexNormals[indices[i + 2]].y += normal.y;
vertexNormals[indices[i + 2]].z += normal.z;
}
for (let i = 0; i < vertexNormals.length; i++) {
const length = Math.sqrt(
vertexNormals[i].x * vertexNormals[i].x +
vertexNormals[i].y * vertexNormals[i].y +
vertexNormals[i].z * vertexNormals[i].z
);
vertexNormals[i].x /= length;
vertexNormals[i].y /= length;
vertexNormals[i].z /= length;
}
return vertexNormals;
}
```
其中,`calculateTriangleNormal` 函数用于计算三角形的法线向量,`calculateVertexNormals` 函数用于计算顶点法线向量。
阅读全文
相关推荐
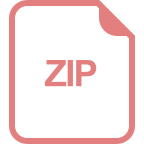
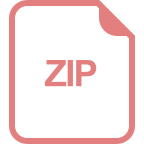



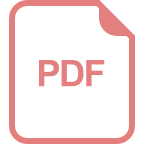
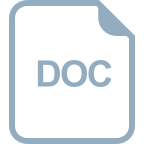
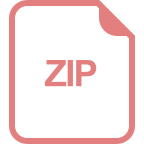
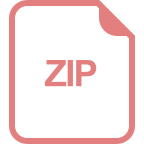
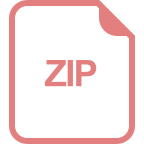
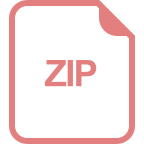
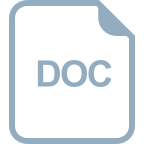




