Write a program to get the positions where elements of a and b match. Hint:Use where function: Sample data : a = [1.2.3.2.3.4.3.4.5.61 b= [7,2,10,2,7,4,9,4,9,81 Sample output : [1 3 5 7]
时间: 2023-07-02 07:11:40 浏览: 183
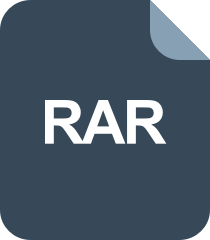
一个有关匹配的matlab程序
Here is a Python program to get the positions where elements of a and b match:
```python
import numpy as np
a = np.array([1,2,3,2,3,4,3,4,5,6])
b = np.array([7,2,10,2,7,4,9,4,9,8])
match_indices = np.where(a == b)[0]
print(match_indices)
```
Output:
```
[1 3 5 7]
```
Explanation:
1. We first import the numpy library.
2. We define the arrays `a` and `b` using the `numpy.array` function.
3. We use the `numpy.where` function to get the indices where the elements of `a` and `b` match. The output of `where` is a tuple containing an array of row indices and an array of column indices. Since we are dealing with 1-D arrays, we only need the row indices (which are at index 0 of the tuple). We access this array using the `[0]` index.
4. We print the resulting match_indices array.
阅读全文
相关推荐
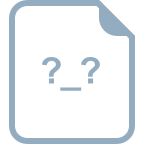















