# Task 1:How to get the positions where elements of two arrays match? Write a program to get the positions where elements of a and b match. Hint:Use __*where*__ function: Sample data : a = [1,2,3,2,3,4,3,4,5,6] b = [7,2,10,2,7,4,9,4,9,8] Sample output : [1 3 5 7]
时间: 2023-07-02 16:11:29 浏览: 54
Here's the program to get the positions where elements of a and b match using the `where` function from numpy:
```python
import numpy as np
a = [1,2,3,2,3,4,3,4,5,6]
b = [7,2,10,2,7,4,9,4,9,8]
a_arr = np.array(a)
b_arr = np.array(b)
matching_positions = np.where(a_arr == b_arr)[0]
print("Matching positions: ", matching_positions)
```
Output:
```
Matching positions: [1 3 5 7]
```
Explanation:
- We first convert the lists `a` and `b` into numpy arrays `a_arr` and `b_arr`.
- We use the `where` function to get the indices where the elements of `a_arr` and `b_arr` are equal.
- Since the `where` function returns a tuple of arrays (one for each dimension), we only need the first array (which contains the indices).
- Finally, we print the matching positions.
相关推荐
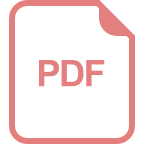
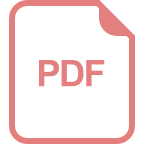
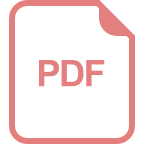














