蛇身为网格的贪吃蛇代码Java
时间: 2023-07-02 11:07:46 浏览: 163
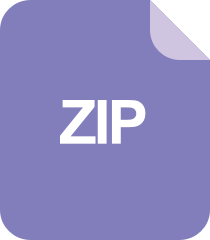
贪吃蛇代码Java
以下是一个简单的Java贪吃蛇游戏代码:
```
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Snake extends JPanel implements Runnable, KeyListener {
private static final long serialVersionUID = 1L;
public static final int WIDTH = 500, HEIGHT = 500;
private Thread thread;
private boolean running = false;
private Point head;
private ArrayList<Point> snake;
private int direction;
private boolean gameOver = false;
private Point food;
private Random r;
public Snake() {
setPreferredSize(new Dimension(WIDTH, HEIGHT));
setBackground(Color.BLACK);
setFocusable(true);
addKeyListener(this);
head = new Point(WIDTH / 2, HEIGHT / 2);
snake = new ArrayList<Point>();
snake.add(head);
direction = 0;
r = new Random();
food = new Point(r.nextInt(WIDTH - 10), r.nextInt(HEIGHT - 10));
start();
}
public void start() {
running = true;
thread = new Thread(this);
thread.start();
}
public void stop() {
running = false;
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public void tick() {
if (!gameOver) {
for (int i = snake.size() - 1; i > 0; i--) {
snake.set(i, new Point(snake.get(i - 1)));
}
switch (direction) {
case 0:
head.y -= 10;
break;
case 1:
head.x += 10;
break;
case 2:
head.y += 10;
break;
case 3:
head.x -= 10;
break;
}
if (head.x < 0 || head.y < 0 || head.x > WIDTH || head.y > HEIGHT) {
gameOver = true;
}
if (head.equals(food)) {
snake.add(new Point(snake.get(snake.size() - 1)));
food.setLocation(r.nextInt(WIDTH - 10), r.nextInt(HEIGHT - 10));
}
for (int i = 1; i < snake.size(); i++) {
if (head.equals(snake.get(i))) {
gameOver = true;
}
}
}
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (!gameOver) {
g.setColor(Color.WHITE);
g.fillRect(food.x, food.y, 10, 10);
g.setColor(Color.GREEN);
for (Point p : snake) {
g.fillRect(p.x, p.y, 10, 10);
}
} else {
g.setColor(Color.WHITE);
g.drawString("Game Over", WIDTH / 2 - 30, HEIGHT / 2);
}
}
public void run() {
while (running) {
tick();
repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
stop();
}
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_UP) {
if (direction != 2) {
direction = 0;
}
} else if (key == KeyEvent.VK_RIGHT) {
if (direction != 3) {
direction = 1;
}
} else if (key == KeyEvent.VK_DOWN) {
if (direction != 0) {
direction = 2;
}
} else if (key == KeyEvent.VK_LEFT) {
if (direction != 1) {
direction = 3;
}
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setTitle("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(new Snake());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
这个代码实现了一个基本的贪吃蛇游戏,使用网格来表示蛇和食物的位置。玩家可以使用上下左右方向键来控制蛇的移动方向,当蛇吃到食物时,它的身体会变长一节。如果蛇撞到墙壁或者自己的身体,游戏就会结束。
阅读全文
相关推荐
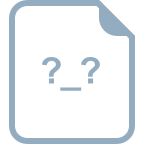
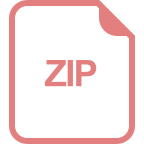
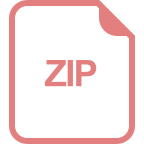
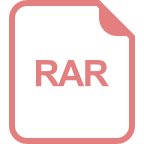
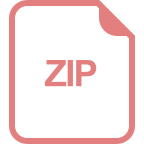
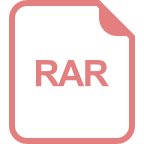
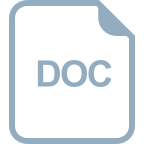
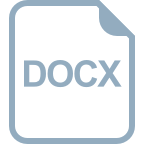
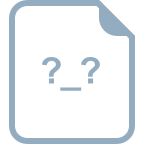
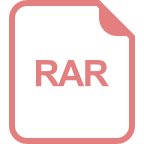
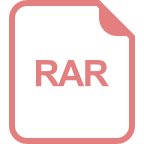
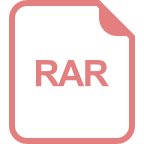
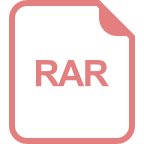
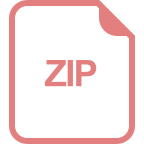
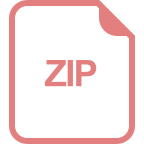
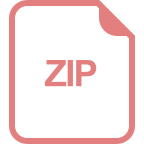
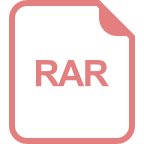