文件浏览框选择指定wav文件并绘制1/3倍频程结果,并封装成函数,最后通过gui界面展示
时间: 2024-02-22 07:56:11 浏览: 155
好的,我来逐步回答你的问题。
首先,关于选择指定wav文件并绘制1/3倍频程结果,可以使用Python中的音频处理库`librosa`来实现。具体步骤如下:
1. 导入`librosa`库和其他必要的库:
```python
import librosa
import numpy as np
import matplotlib.pyplot as plt
```
2. 使用`librosa`库中的`load`函数加载指定的wav文件:
```python
y, sr = librosa.load('path/to/wav/file.wav')
```
其中,`y`是音频信号的时间序列,`sr`是采样率。
3. 计算1/3倍频程的上下限:
```python
fmin = 20
fmax = sr // 2
n_fft = 2048
hop_length = 512
fmin_idx = librosa.core.fft_frequencies(sr=sr, n_fft=n_fft).searchsorted(fmin)
fmax_idx = librosa.core.fft_frequencies(sr=sr, n_fft=n_fft).searchsorted(fmax)
```
其中,`n_fft`是FFT的窗口大小,`hop_length`是窗口移动的步长。
4. 计算1/3倍频程的能量谱:
```python
S = librosa.stft(y, n_fft=n_fft, hop_length=hop_length)
S = np.abs(S)
S_third = librosa.feature.melspectrogram(S=S, sr=sr, n_fft=n_fft, hop_length=hop_length, fmin=fmin, fmax=fmax, n_mels=128)
S_third_db = librosa.power_to_db(S_third, ref=np.max)
S_third_db = S_third_db[fmin_idx:fmax_idx, :]
```
其中,`S`是FFT变换后的频谱矩阵,`S_third`是1/3倍频程的能量谱,`S_third_db`是能量谱转换为dB值后的结果。
5. 绘制1/3倍频程的能量谱图:
```python
plt.figure(figsize=(10, 4))
librosa.display.specshow(S_third_db, x_axis='time', y_axis='mel', sr=sr, fmin=fmin, fmax=fmax)
plt.colorbar(format='%+2.0f dB')
plt.title('1/3 Octave spectrogram')
plt.tight_layout()
plt.show()
```
以上就是实现选择指定wav文件并绘制1/3倍频程结果的全部步骤。
接下来,关于封装成函数,可以将以上的代码封装成一个函数,如下所示:
```python
def plot_1_3_octave_spectrogram(file_path):
y, sr = librosa.load(file_path)
fmin = 20
fmax = sr // 2
n_fft = 2048
hop_length = 512
fmin_idx = librosa.core.fft_frequencies(sr=sr, n_fft=n_fft).searchsorted(fmin)
fmax_idx = librosa.core.fft_frequencies(sr=sr, n_fft=n_fft).searchsorted(fmax)
S = librosa.stft(y, n_fft=n_fft, hop_length=hop_length)
S = np.abs(S)
S_third = librosa.feature.melspectrogram(S=S, sr=sr, n_fft=n_fft, hop_length=hop_length, fmin=fmin, fmax=fmax, n_mels=128)
S_third_db = librosa.power_to_db(S_third, ref=np.max)
S_third_db = S_third_db[fmin_idx:fmax_idx, :]
plt.figure(figsize=(10, 4))
librosa.display.specshow(S_third_db, x_axis='time', y_axis='mel', sr=sr, fmin=fmin, fmax=fmax)
plt.colorbar(format='%+2.0f dB')
plt.title('1/3 Octave spectrogram')
plt.tight_layout()
plt.show()
```
最后,关于通过gui界面展示,可以使用Python中的GUI库`Tkinter`来实现。具体步骤如下:
1. 导入`Tkinter`库和其他必要的库:
```python
import tkinter as tk
from tkinter import filedialog
```
2. 创建一个窗口,并添加一个按钮,用于选择要处理的wav文件:
```python
root = tk.Tk()
root.title('1/3 Octave spectrogram')
def choose_file():
file_path = filedialog.askopenfilename()
plot_1_3_octave_spectrogram(file_path)
btn = tk.Button(root, text='Choose a file', command=choose_file)
btn.pack()
root.mainloop()
```
其中,`choose_file`函数用于选择要处理的wav文件,并调用之前封装好的函数`plot_1_3_octave_spectrogram`来绘制1/3倍频程的能量谱图。`btn`按钮则用于触发选择文件的操作。
以上就是通过GUI界面展示的全部步骤。
希望我的回答能够帮到你!如果你还有其他问题或需要进一步的帮助,请随时提出。
阅读全文
相关推荐
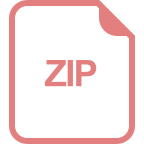
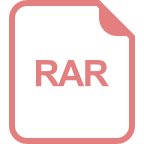
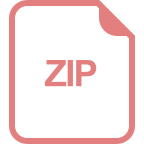
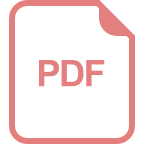
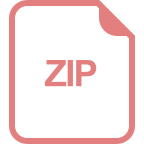
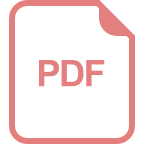
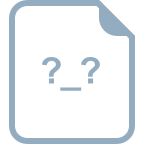
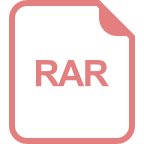
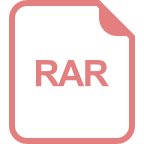
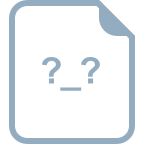
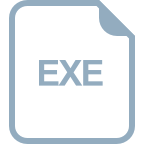
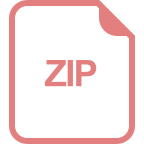
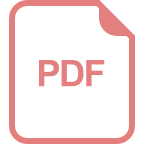