双目相机立体校正python
时间: 2023-05-08 20:00:43 浏览: 165
双目相机立体校正是指消除双目相机在拍摄场景时由于光学偏差而导致图像的不一致性,从而得到更加精准的深度信息。在Python中,可以使用OpenCV等图像处理库来实现双目相机立体校正。
首先,需要对每个相机进行单独的校正,即摄像机内参矩阵和畸变参数的计算。然后,通过选择相同场景点在左右相机中的对应像素点,计算出两个相机的外参矩阵。接着,使用cv2.stereoRectify()函数计算出左右相机的映射矩阵和去畸变之后的图像。
在运行程序时,需要加载左右相机的图像,并通过cv2.imshow()函数显示校正之后的图像。校正后的图像将具有更好的空间一致性和更加准确的深度信息。
需要注意的是,双目相机立体校正的效果受到多种因素的影响,如相机精度、场景复杂度等。因此,在实际应用时,还需要对算法进行优化和调试,以达到最佳效果。
相关问题
双目图像校正python
要进行双目图像校正的话,你可以使用OpenCV库来实现。下面是一个使用Python进行双目图像校正的示例代码:
```python
import cv2
import numpy as np
# 读取左右相机的图像
img_left = cv2.imread('left_image.jpg')
img_right = cv2.imread('right_image.jpg')
# 定义相机的内参和畸变系数
camera_matrix = np.array([[fx, 0, cx],
[0, fy, cy],
[0, 0, 1]])
distortion_coeffs = np.array([k1, k2, p1, p2, k3])
# 获取图像的大小
image_size = (img_left.shape[1], img_left.shape[0])
# 进行双目校正
retval, _, _, _, _, R, T, E, F = cv2.stereoCalibrate(
object_points, image_points_left, image_points_right,
camera_matrix, distortion_coeffs,
camera_matrix, distortion_coeffs,
image_size)
# 计算校正变换
R1, R2, P1, P2, Q, _, _ = cv2.stereoRectify(
camera_matrix, distortion_coeffs,
camera_matrix, distortion_coeffs,
image_size, R, T)
# 根据校正变换进行图像校正
map1_left, map2_left = cv2.initUndistortRectifyMap(
camera_matrix, distortion_coeffs, R1, P1, image_size, cv2.CV_16SC2)
map1_right, map2_right = cv2.initUndistortRectifyMap(
camera_matrix, distortion_coeffs, R2, P2, image_size, cv2.CV_16SC2)
img_left_rectified = cv2.remap(img_left, map1_left, map2_left, cv2.INTER_LINEAR)
img_right_rectified = cv2.remap(img_right, map1_right, map2_right, cv2.INTER_LINEAR)
# 显示校正后的图像
cv2.imshow("Left Rectified", img_left_rectified)
cv2.imshow("Right Rectified", img_right_rectified)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这段代码中,你需要根据实际情况替换左右相机图像的文件路径、相机的内参和畸变系数,以及校正所需的点云和图像点。然后,代码会根据输入的参数进行双目图像校正,并显示校正后的图像。
请确保已经安装了OpenCV库,并且将左右相机的图像文件放置在正确的路径下。另外,根据你的实际需求,你可能还需要做一些额外的处理,比如立体匹配等。
双目相机标定 python
双目相机标定可以使用OpenCV库中的函数进行实现。具体步骤如下:
1. 拍摄一组双目图像,保证左右相机视角有一定重叠区域。
2. 提取每张图像中的角点,可以使用OpenCV中的findChessboardCorners函数。
3. 对每张图像中提取到的角点进行亚像素精确化,可以使用cornerSubPix函数。
4. 根据左右相机的内参矩阵和外参矩阵,计算出双目相机的基础矩阵和投影矩阵,可以使用stereoCalibrate函数。
5. 对双目相机进行立体校正,可以使用stereoRectify函数。
相关推荐
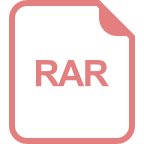
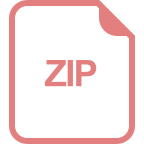












