定义一个抽象类Shape,该类有抽象方法area,圆形、矩形、梯形等均是一种Shape,也都有自己求area方法,定义这些类并写测试类进行测试。Java
时间: 2024-10-19 11:13:20 浏览: 8
在Java中,你可以创建一个抽象类`Shape`来定义一个形状的基本属性和行为。抽象类包含抽象方法,这意味着它的实例化是不可能的,它主要用于被其他类继承和扩展。下面是一个简单的例子:
```java
// 定义抽象类 Shape
abstract class Shape {
// 抽象方法 area,需要由子类覆盖实现
abstract double getArea();
// 其他通用的方法或属性可以放在这里
public String getName() {
return getClass().getSimpleName();
}
}
// 实现 Shape 的子类 - 圆形 Circle
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
double getArea() {
return Math.PI * radius * radius;
}
}
// 实现 Shape 的子类 - 矩形 Rectangle
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
double getArea() {
return width * height;
}
}
// 实现 Shape 的子类 - 梯形 Trapezoid
class Trapezoid extends Shape {
private double base1;
private double base2;
private double height;
public Trapezoid(double base1, double base2, double height) {
this.base1 = base1;
this.base2 = base2;
this.height = height;
}
@Override
double getArea() {
return (base1 + base2) * height / 2;
}
}
// 测试类 TestShape
public class TestShape {
public static void main(String[] args) {
Circle circle = new Circle(5);
System.out.println(circle.getName() + " 的面积: " + circle.getArea());
Rectangle rectangle = new Rectangle(4, 6);
System.out.println(rectangle.getName() + " 的面积: " + rectangle.getArea());
Trapezoid trapezoid = new Trapezoid(3, 7, 4);
System.out.println(trapezoid.getName() + " 的面积: " + trapezoid.getArea());
// 这里演示了多态性,虽然传递的是 Shape 类型的引用,但根据实际操作的对象,会调用相应子类的 getArea 方法
Shape shape = new Circle(2);
System.out.println(shape.getName() + " 的面积: " + shape.getArea());
}
}
```
在这个例子中,`TestShape`类通过`Shape`类型的引用来调用各个子类的方法,这就是多态的具体体现。
阅读全文
相关推荐
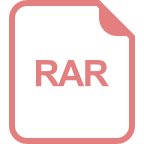
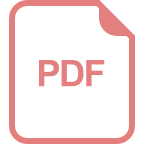
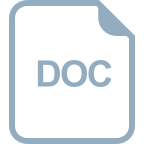















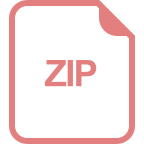