这里面的{hash:true,empty:true}有什么意义
时间: 2024-04-21 08:24:29 浏览: 12
根据上面的代码片段,`{hash:true,empty:true}` 是作第二个参数传递给 `serialize` 函数的一个配置对象。
在这个上下文中,`{hash:true,empty:true}` 可能表示了一些序列化选项,用于指定序列化的行为。具体来说,可能有以下解释:
- `hash:true`:表示在序列化过程中包含对象的哈希值。哈希值是一个唯一的标识符,用于标识对象的内容。这个选项可能会在序列化后的字符串中包含一个用于表示对象哈希值的字段。
- `empty:true`:表示允许序列化空属性。如果对象中存在空属性(属性值为 undefined 或 null),那么这个选项可能会让序列化后的结果中保留这些空属性。
请注意,上述解释只是对可能含义的猜测,具体取决于 `serialize` 函数的实现和调用者的意图。要准确理解 `{hash:true,empty:true}` 的含义,你需要查阅相关文档或查看 `serialize` 函数的实现代码。
相关问题
const { id, bookname, author, publisher } = serialize(editForm,{hash:true,empty:true})
这是一个解构赋值的语法,它从 `serialize(editForm,{hash:true,empty:true})` 的结果中提取出 `id`、`bookname`、`author` 和 `publisher` 属性的值,并将它们分别赋值给对应的变量。
假设 `serialize(editForm,{hash:true,empty:true})` 的结果是一个对象,且该对象具有 `id`、`bookname`、`author` 和 `publisher` 属性,那么上述解构赋值语句将会将这些属性的值赋值给对应的变量。
例如,如果 `serialize(editForm,{hash:true,empty:true})` 的结果是 `{ id: 1, bookname: 'JavaScript Basics', author: 'John Doe', publisher: 'CSDN Press' }`,那么解构赋值后,`id` 的值将为 1,`bookname` 的值将为 'JavaScript Basics',`author` 的值将为 'John Doe',`publisher` 的值将为 'CSDN Press'。
这里面的serialize是什么意思
`serialize` 是一个函数或方法,用于将对象转换为字符串或其他序列化格式,以便在不同的环境中进行传输或存储。在这个上下文中,`serialize` 函数可能是一个自定义函数或来自某个库或框架。
根据你提供的代码片段,我们可以猜测 `serialize` 函数接受两个参数:`editForm` 对象和一个配置对象 `{hash:true,empty:true}`。它可能根据配置选项将 `editForm` 对象序列化为一个字符串,并返回一个包含序列化结果的对象。
由于上述代码片段缺少 `serialize` 函数的具体实现细节,我们无法确定它的确切行为。你需要查看代码中 `serialize` 函数的定义或者提供更多关于 `serialize` 函数的上下文信息,以便更好地理解其含义和功能。
相关推荐
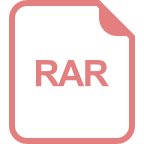
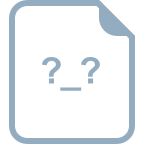
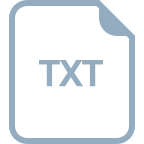













