建立图并输出图并广度优先遍历和深度优先遍历
时间: 2023-12-18 10:29:26 浏览: 108
以下是JavaScript树的深度优先遍历和广度优先遍历算法的实现:
```javascript
// 定义树节点
class TreeNode {
constructor(val) {
this.val = val;
this.left = null;
this.right = null;
}
}
// 深度优先遍历(递归)
function dfs(root) {
if (!root) return;
console.log(root.val);
dfs(root.left);
dfs(root.right);
}
// 深度优先遍历(非递归)
function dfs2(root) {
if (!root) return;
const stack = [root];
while (stack.length) {
const node = stack.pop();
console.log(node.val);
if (node.right) stack.push(node.right);
if (node.left) stack.push(node.left);
}
}
// 广度优先遍历
function bfs(root) {
if (!root) return;
const queue = [root];
while (queue.length) {
const node = queue.shift();
console.log(node.val);
if (node.left) queue.push(node.left);
if (node.right) queue.push(node.right);
}
}
// 创建一棵树
const root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
root.right.left = new TreeNode(6);
root.right.right = new TreeNode(7);
// 深度优先遍历
dfs(root); // 输出:1 2 4 5 3 6 7
// 深度优先遍历(非递归)
dfs2(root); // 输出:1 3 7 6 2 5 4
// 广度优先遍历
bfs(root); // 输出:1 2 3 4 5 6 7
```
以下是以邻接表为存储结构,实现连通无向图的深度优先和广度优先遍历的实现:
```python
# 定义图节点
class GraphNode:
def __init__(self, val):
self.val = val
self.neighbors = []
# 深度优先遍历
def dfs(node):
if not node:
return
visited = set()
stack = [node]
while stack:
cur = stack.pop()
if cur not in visited:
visited.add(cur)
print(cur.val)
for neighbor in cur.neighbors:
stack.append(neighbor)
# 广度优先遍历
def bfs(node):
if not node:
return
visited = set()
queue = [node]
while queue:
cur = queue.pop(0)
if cur not in visited:
visited.add(cur)
print(cur.val)
for neighbor in cur.neighbors:
queue.append(neighbor)
# 创建一个图
node1 = GraphNode(1)
node2 = GraphNode(2)
node3 = GraphNode(3)
node4 = GraphNode(4)
node5 = GraphNode(5)
node6 = GraphNode(6)
node1.neighbors = [node2, node3]
node2.neighbors = [node1, node4, node5]
node3.neighbors = [node1, node6]
node4.neighbors = [node2]
node5.neighbors = [node2, node6]
node6.neighbors = [node3, node5]
# 深度优先遍历
dfs(node1) # 输出:1 3 6 5 2 4
# 广度优先遍历
bfs(node1) # 输出:1 2 3 4 5 6
```
阅读全文
相关推荐
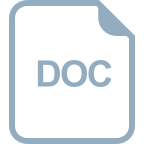
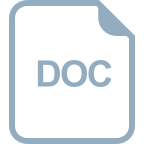
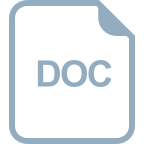



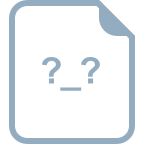
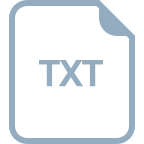






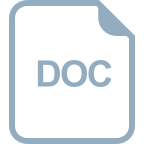
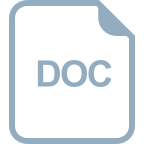
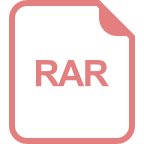
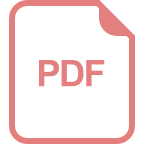