用C++写某一点到线段的最短距离
时间: 2023-10-14 22:04:22 浏览: 55
假设点为P,线段的两端点为A和B。首先需要求出点P到线段AB所在直线的垂足点H,然后判断垂足点H是否在线段AB上。如果在,则垂足点就是点P到线段AB的最短距离;如果不在,则最短距离为点P到线段AB两端点的距离的最小值。
以下是具体实现代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
struct Point {
double x, y;
};
// 计算两点间距离
double calcDistance(Point a, Point b) {
return sqrt(pow(a.x - b.x, 2) + pow(a.y - b.y, 2));
}
// 计算点P到直线AB的垂足点H
Point calcPerpendicular(Point p, Point a, Point b) {
double k = ((p.x - a.x) * (b.x - a.x) + (p.y - a.y) * (b.y - a.y)) / pow(calcDistance(a, b), 2);
double hx = a.x + k * (b.x - a.x);
double hy = a.y + k * (b.y - a.y);
return Point{hx, hy};
}
// 判断垂足点H是否在线段AB上
bool isOnSegment(Point h, Point a, Point b) {
return min(a.x, b.x) <= h.x && h.x <= max(a.x, b.x) && min(a.y, b.y) <= h.y && h.y <= max(a.y, b.y);
}
// 计算点P到线段AB的最短距离
double calcShortestDistance(Point p, Point a, Point b) {
Point h = calcPerpendicular(p, a, b);
if (isOnSegment(h, a, b)) {
return calcDistance(p, h);
} else {
return min(calcDistance(p, a), calcDistance(p, b));
}
}
int main() {
Point p{1, 1};
Point a{0, 0};
Point b{2, 0};
cout << calcShortestDistance(p, a, b) << endl; // 输出 1
return 0;
}
```
以上代码中,假设点P为(1,1),线段的两端点分别为A(0,0)和B(2,0),输出结果为1,表示点P到线段AB的最短距离为1。
相关推荐
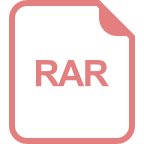
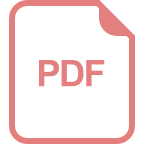










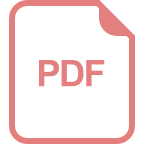
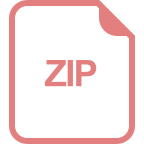
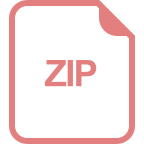