用C/C++写一个图地铁最短路径项目,算法用Dijskra
时间: 2024-04-22 09:24:48 浏览: 8
当然可以!以下是一个用C++实现Dijkstra算法的图地铁最短路径项目的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
#define INF 9999
// 图的节点类
class Node {
public:
int id;
int distance;
bool visited;
vector<pair<int, int>> neighbors; // 邻居节点及边的权重
Node(int _id) {
id = _id;
distance = INF;
visited = false;
}
void addNeighbor(int neighbor, int weight) {
neighbors.push_back(make_pair(neighbor, weight));
}
};
// 图类
class Graph {
public:
vector<Node> nodes;
// 添加节点
void addNode(Node node) {
nodes.push_back(node);
}
// 添加边
void addEdge(int src, int dest, int weight) {
nodes[src].addNeighbor(dest, weight);
nodes[dest].addNeighbor(src, weight);
}
// Dijkstra算法
void shortestPath(int start) {
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<pair<int, int>>> pq;
// 初始化起点
nodes[start].distance = 0;
pq.push(make_pair(0, start));
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
if (nodes[u].visited) continue;
nodes[u].visited = true;
for (auto neighbor : nodes[u].neighbors) {
int v = neighbor.first;
int weight = neighbor.second;
if (nodes[v].distance > nodes[u].distance + weight) {
nodes[v].distance = nodes[u].distance + weight;
pq.push(make_pair(nodes[v].distance, v));
}
}
}
}
};
int main() {
Graph graph;
// 添加节点
for (int i = 0; i < 6; i++) {
Node node(i);
graph.addNode(node);
}
// 添加边
graph.addEdge(0, 1, 7);
graph.addEdge(0, 2, 9);
graph.addEdge(0, 5, 14);
graph.addEdge(1, 2, 10);
graph.addEdge(1, 3, 15);
graph.addEdge(2, 3, 11);
graph.addEdge(2, 5, 2);
graph.addEdge(3, 4, 6);
graph.addEdge(4, 5, 9);
int start = 0;
graph.shortestPath(start);
// 输出最短路径
for (int i = 0; i < graph.nodes.size(); i++) {
cout << "最短路径从节点 " << start << " 到节点 " << i << " 的距离为:" << graph.nodes[i].distance << endl;
}
return 0;
}
```
上述代码实现了一个简单的图类和节点类,使用 Dijkstra 算法计算了图中每个节点到起点的最短路径。你可以根据自己的需求进行修改和扩展。注意,这只是一个简单的示例,实际项目中可能需要更复杂的数据结构和算法来表示和计算地铁线路。
相关推荐
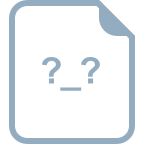
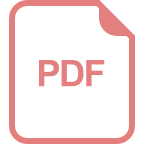
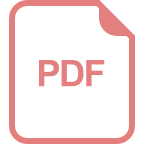
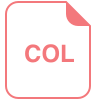

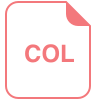
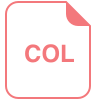










