如何用纯C语言在Linux环境下开发一个串口通信程序,用于数据的接收和发送?
时间: 2024-10-20 22:05:06 浏览: 104
在Linux环境下用纯C语言开发串口通信程序,通常会使用系统库`termios.h`和`unistd.h`,这两个头文件提供了处理串口输入输出的功能。以下是简单的步骤:
1. **包含头文件**:
```c
#include <stdio.h>
#include <fcntl.h> // 对于file descriptor操作
#include <termios.h>
```
2. **打开串口**:
使用`open()`函数打开串口设备(例如 `/dev/ttyS0` 或 `"/dev/ttyUSB0"`),并获取文件描述符(fd)。
```c
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("Opening serial port failed");
exit(1);
}
```
3. **设置串口参数**:
使用`tcgetattr()`获取当前模式,然后用`tcsetattr()`设置模式,比如波特率、数据位、停止位和校验位等,通过`struct termios`结构体来配置。
```c
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600bps
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL | CREAD; // 允许本地模式并允许读取
options.c_iflag &= ~IGNBRK; // 关闭忽略break字符
options.c_oflag &= ~PARENB; // 禁止奇偶校验
options.c_cflag &= ~CSIZE; // 清除数据位大小标志
options.c_cflag |= CS8; // 设置8位数据位
options.c_stopb = 1; // 设置1位停止位
```
4. **数据收发**:
你可以使用`read()`和`write()`来分别从串口接收和发送数据。
```c
char buffer[128];
ssize_t bytes_received = read(fd, buffer, sizeof(buffer));
if (bytes_received > 0) {
printf("Received data: %s\n", buffer);
}
write(fd, "Hello from computer!", strlen("Hello from computer!"));
```
5. **关闭串口**:
最后记得用`close()`函数关闭文件描述符。
```c
close(fd);
```
阅读全文
相关推荐
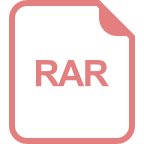
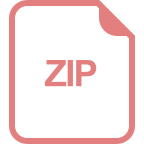
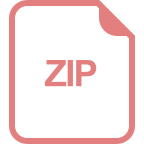















