写一个STM32的HC-SR04测距函数
时间: 2023-05-12 16:03:25 浏览: 107
好的,以下是一个基于STM32的HC-SR04测距函数的示例代码:
```
#include "stm32f10x.h"
#define TRIG_PIN GPIO_Pin_0
#define ECHO_PIN GPIO_Pin_1
#define TRIG_PORT GPIOA
#define ECHO_PORT GPIOA
void delay_us(uint32_t us)
{
uint32_t i;
for(i=0; i<us*8; i++);
}
float hc_sr04_measure_distance(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_ICInitTypeDef TIM_ICInitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
float distance;
uint32_t pulse_width;
uint32_t timeout;
// Enable GPIOA and TIM2 clocks
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
// Configure TRIG pin as output
GPIO_InitStructure.GPIO_Pin = TRIG_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(TRIG_PORT, &GPIO_InitStructure);
// Configure ECHO pin as input
GPIO_InitStructure.GPIO_Pin = ECHO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD;
GPIO_Init(ECHO_PORT, &GPIO_InitStructure);
// Configure TIM2 for input capture mode
TIM_TimeBaseStructure.TIM_Period = 0xFFFF;
TIM_TimeBaseStructure.TIM_Prescaler = 72 - 1;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
TIM_ICInitStructure.TIM_Channel = TIM_Channel_2;
TIM_ICInitStructure.TIM_ICPolarity = TIM_ICPolarity_Rising;
TIM_ICInitStructure.TIM_ICSelection = TIM_ICSelection_DirectTI;
TIM_ICInitStructure.TIM_ICPrescaler = TIM_ICPSC_DIV1;
TIM_ICInitStructure.TIM_ICFilter = 0;
TIM_ICInit(TIM2, &TIM_ICInitStructure);
// Enable TIM2 interrupt
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
// Generate 10us pulse on TRIG pin
GPIO_SetBits(TRIG_PORT, TRIG_PIN);
delay_us(10);
GPIO_ResetBits(TRIG_PORT, TRIG_PIN);
// Wait for ECHO pin to go high
timeout = 0;
while(!GPIO_ReadInputDataBit(ECHO_PORT, ECHO_PIN))
{
if(timeout++ > 10000)
return -1;
}
// Start TIM2
TIM_Cmd(TIM2, ENABLE);
// Wait for ECHO pin to go low
timeout = 0;
while(GPIO_ReadInputDataBit(ECHO_PORT, ECHO_PIN))
{
if(timeout++ > 10000)
return -1;
}
// Stop TIM2
TIM_Cmd(TIM2, DISABLE);
// Calculate pulse width
pulse_width = TIM_GetCapture2(TIM2);
// Calculate distance in cm
distance = pulse_width * 0.034 / 2;
return distance;
}
void TIM2_IRQHandler(void)
{
TIM_ClearITPendingBit(TIM2, TIM_IT_CC2);
}
```
这个函数使用STM32的定时器和输入捕获功能来测量HC-SR04超声波传感器返回的脉冲宽度,并计算出距离。
阅读全文
相关推荐
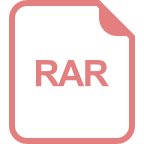



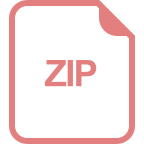
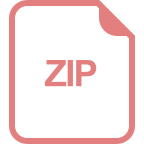
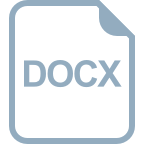
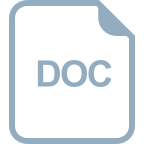





