python写个俄罗斯方块游戏
时间: 2023-08-28 08:06:27 浏览: 63
好的,下面是一个简单的俄罗斯方块游戏的Python实现:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (128, 128, 128)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
CYAN = (0, 255, 255)
MAGENTA = (255, 0, 255)
YELLOW = (255, 255, 0)
# 游戏区域大小
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
# 方块大小
BLOCK_SIZE = 20
# 方块类型定义
BLOCK_TYPES = [
[
[1, 1, 1],
[0, 1, 0],
],
[
[2, 2],
[2, 2],
],
[
[0, 3, 3],
[3, 3, 0],
],
[
[4, 0, 0],
[4, 4, 4],
],
[
[0, 0, 5],
[5, 5, 5],
],
[
[6, 6, 0],
[0, 6, 6],
],
[
[0, 7, 7],
[7, 7, 0],
],
]
# 游戏区域
game_area = []
# 初始化游戏区域
def init_game_area():
global game_area
game_area = [[0] * (SCREEN_WIDTH // BLOCK_SIZE) for i in range(SCREEN_HEIGHT // BLOCK_SIZE)]
# 绘制游戏区域
def draw_game_area(screen):
for y in range(len(game_area)):
for x in range(len(game_area[y])):
if game_area[y][x] == 0:
pygame.draw.rect(screen, GRAY, [x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE], 1)
else:
pygame.draw.rect(screen, get_block_color(game_area[y][x]), [x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE])
# 生成随机方块
def generate_block():
return random.choice(BLOCK_TYPES)
# 获取方块颜色
def get_block_color(block_type):
if block_type == 1:
return CYAN
elif block_type == 2:
return YELLOW
elif block_type == 3:
return MAGENTA
elif block_type == 4:
return BLUE
elif block_type == 5:
return GREEN
elif block_type == 6:
return RED
elif block_type == 7:
return WHITE
# 方块移动
def move_block(block, dx, dy):
for y in range(len(block)):
for x in range(len(block[y])):
if block[y][x] != 0:
game_area[y + dy][x + dx] = block[y][x]
# 方块旋转
def rotate_block(block):
return [[block[y][x] for y in range(len(block))] for x in range(len(block[0]) - 1, -1, -1)]
# 检查是否可以移动方块
def check_move_block(block, dx, dy):
for y in range(len(block)):
for x in range(len(block[y])):
if block[y][x] != 0:
if y + dy >= len(game_area) or x + dx < 0 or x + dx >= len(game_area[y]) or game_area[y + dy][x + dx] != 0:
return False
return True
# 检查是否可以旋转方块
def check_rotate_block(block):
rotated_block = rotate_block(block)
for y in range(len(rotated_block)):
for x in range(len(rotated_block[y])):
if rotated_block[y][x] != 0:
if y >= len(game_area) or x < 0 or x >= len(game_area[y]) or game_area[y][x] != 0:
return False
return True
# 消除满行
def remove_full_rows():
rows_to_remove = []
for y in range(len(game_area)):
if all(game_area[y][x] != 0 for x in range(len(game_area[y]))):
rows_to_remove.append(y)
for y in rows_to_remove:
for i in range(y, 1, -1):
game_area[i] = game_area[i - 1]
game_area[0] = [0] * len(game_area[0])
# 游戏主循环
def main_loop():
# 初始化游戏区域
init_game_area()
# 生成第一个方块
block = generate_block()
block_x = (len(game_area[0]) - len(block[0])) // 2
block_y = 0
# 游戏主循环
clock = pygame.time.Clock()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
# 处理方块移动和旋转
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and check_move_block(block, block_x - 1, block_y):
block_x -= 1
if keys[pygame.K_RIGHT] and check_move_block(block, block_x + 1, block_y):
block_x += 1
if keys[pygame.K_DOWN] and check_move_block(block, block_x, block_y + 1):
block_y += 1
if keys[pygame.K_UP] and check_rotate_block(block):
block = rotate_block(block)
# 检查是否可以移动方块
if check_move_block(block, block_x, block_y + 1):
block_y += 1
else:
move_block(block, block_x, block_y)
remove_full_rows()
block = generate_block()
block_x = (len(game_area[0]) - len(block[0])) // 2
block_y = 0
# 绘制游戏区域和方块
screen.fill(BLACK)
draw_game_area(screen)
for y in range(len(block)):
for x in range(len(block[y])):
if block[y][x] != 0:
pygame.draw.rect(screen, get_block_color(block[y][x]), [(block_x + x) * BLOCK_SIZE, (block_y + y) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE])
# 更新屏幕
pygame.display.flip()
# 控制帧率
clock.tick(10)
# 创建窗口
screen = pygame.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
# 运行游戏
main_loop()
# 退出Pygame
pygame.quit()
```
这个实现并不完整,还可以添加更多的功能和优化。
阅读全文
相关推荐
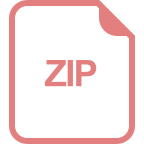











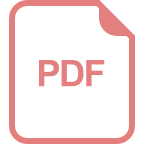
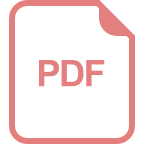
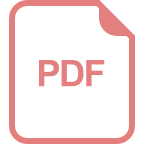
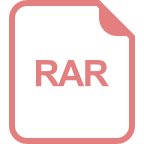
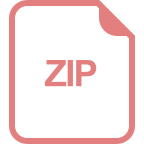