用python写个俄罗斯方块的代码
时间: 2023-11-13 20:26:03 浏览: 71
好的,以下是一个用 Python 实现俄罗斯方块游戏的示例代码,基于 Pygame 游戏开发库:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 定义游戏区域的大小
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
# 定义方块的大小
BLOCK_SIZE = 20
# 定义不同形状的方块
SHAPES = [
[[1, 1, 1], [0, 1, 0]],
[[2, 2], [2, 2]],
[[3, 3, 0], [0, 3, 3]],
[[0, 4, 4], [4, 4, 0]],
[[5, 5, 5, 5]],
[[6, 6, 6], [0, 0, 6]],
[[7, 7, 7], [7, 0, 0], [0, 7, 0]]
]
# 定义方块的颜色
COLORS = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255),
(128, 128, 128)
]
# 创建游戏区域的 Surface
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 设置游戏窗口的标题
pygame.display.set_caption("俄罗斯方块")
# 创建游戏时钟
clock = pygame.time.Clock()
# 定义方块类
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
self.color = random.randint(1, len(COLORS) - 1)
def draw(self, surface):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] != 0:
pygame.draw.rect(surface, COLORS[self.color], (self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
def move_down(self):
self.y += BLOCK_SIZE
def move_left(self):
self.x -= BLOCK_SIZE
def move_right(self):
self.x += BLOCK_SIZE
def rotate(self):
self.shape = [[self.shape[j][i] for j in range(len(self.shape))] for i in range(len(self.shape[0]) - 1, -1, -1)]
# 定义游戏主循环
def main():
# 创建当前方块和下一个方块
cur_block = Block(SCREEN_WIDTH // 2 - BLOCK_SIZE * 2, 0, random.choice(SHAPES))
next_block = Block(SCREEN_WIDTH - SCREEN_WIDTH // 4, SCREEN_HEIGHT // 4, random.choice(SHAPES))
# 定义游戏循环的变量
running = True
game_over = False
score = 0
# 定义游戏循环
while running:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if not game_over:
if event.key == pygame.K_LEFT:
cur_block.move_left()
if check_collision(cur_block):
cur_block.move_right()
elif event.key == pygame.K_RIGHT:
cur_block.move_right()
if check_collision(cur_block):
cur_block.move_left()
elif event.key == pygame.K_DOWN:
cur_block.move_down()
if check_collision(cur_block):
cur_block.move_up()
add_to_grid(cur_block)
cur_block = next_block
next_block = Block(SCREEN_WIDTH - SCREEN_WIDTH // 4, SCREEN_HEIGHT // 4, random.choice(SHAPES))
if check_collision(cur_block):
game_over = True
elif event.key == pygame.K_UP:
cur_block.rotate()
if check_collision(cur_block):
cur_block.rotate()
cur_block.rotate()
cur_block.rotate()
# 绘制游戏界面
screen.fill(COLORS[0])
draw_grid(screen)
cur_block.draw(screen)
next_block.draw(screen)
draw_score(screen, score)
if game_over:
draw_game_over(screen)
# 更新游戏时钟
clock.tick(10)
# 移动当前方块
cur_block.move_down()
if check_collision(cur_block):
cur_block.move_up()
add_to_grid(cur_block)
cur_block = next_block
next_block = Block(SCREEN_WIDTH - SCREEN_WIDTH // 4, SCREEN_HEIGHT // 4, random.choice(SHAPES))
if check_collision(cur_block):
game_over = True
# 检测是否可以消除行
lines_cleared = 0
for i in range(len(grid)):
if all(grid[i]):
lines_cleared += 1
for j in range(i, 0, -1):
grid[j] = grid[j-1].copy()
grid[0] = [0] * (SCREEN_WIDTH // BLOCK_SIZE)
# 更新得分
score += lines_cleared * 100
# 更新游戏显示
pygame.display.flip()
# 退出 Pygame
pygame.quit()
# 定义检测方块是否碰撞的函数
def check_collision(block):
if block.x < 0 or block.x + len(block.shape[0]) * BLOCK_SIZE > SCREEN_WIDTH:
return True
if block.y + len(block.shape) * BLOCK_SIZE > SCREEN_HEIGHT:
return True
for i in range(len(block.shape)):
for j in range(len(block.shape[i])):
if block.shape[i][j] != 0 and grid[(block.y // BLOCK_SIZE) + i][(block.x // BLOCK_SIZE) + j] != 0:
return True
return False
# 定义将方块添加到游戏区域的函数
def add_to_grid(block):
for i in range(len(block.shape)):
for j in range(len(block.shape[i])):
if block.shape[i][j] != 0:
grid[(block.y // BLOCK_SIZE) + i][(block.x // BLOCK_SIZE) + j] = block.color
# 定义绘制游戏区域的函数
def draw_grid(surface):
for i in range(len(grid)):
for j in range(len(grid[i])):
pygame.draw.rect(surface, COLORS[grid[i][j]], (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE), 1)
# 定义绘制得分的函数
def draw_score(surface, score):
font = pygame.font.SysFont(None, 30)
text = font.render(f"Score: {score}", True, COLORS[7])
surface.blit(text, (10, 10))
# 定义绘制游戏结束的函数
def draw_game_over(surface):
font = pygame.font.SysFont(None, 60)
text = font.render("Game Over", True, COLORS[1])
surface.blit(text, (SCREEN_WIDTH // 2 - text.get_width() // 2, SCREEN_HEIGHT // 2 - text.get_height() // 2))
# 创建游戏区域的网格
grid = [[0] * (SCREEN_WIDTH // BLOCK_SIZE) for _ in range(SCREEN_HEIGHT // BLOCK_SIZE)]
# 运行游戏主循环
if __name__ == "__main__":
main()
```
这个示例代码实现了一个基本的俄罗斯方块游戏,包括方块的生成、下落、移动、旋转、消除、得分计算等功能。您可以根据自己的需求进行修改和优化。
相关推荐
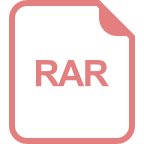
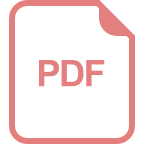












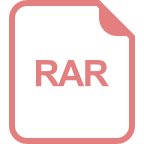