图片批量化进行弹性形变 仿射变换 旋转变换python代码
时间: 2023-10-26 11:10:21 浏览: 45
以下是使用Python进行图片批量化进行弹性形变、仿射变换、旋转变换的示例代码:
弹性形变:
```python
import numpy as np
import cv2
import os
def elastic_transform(image, alpha, sigma, alpha_affine, random_state=None):
if random_state is None:
random_state = np.random.RandomState(None)
shape = image.shape
shape_size = shape[:2]
# Random affine
center_square = np.float32(shape_size) // 2
square_size = min(shape_size) // 3
pts1 = np.float32([center_square + square_size, [center_square[0]+square_size, center_square[1]-square_size], center_square - square_size])
pts2 = pts1 + random_state.uniform(-alpha_affine, alpha_affine, size=pts1.shape).astype(np.float32)
M = cv2.getAffineTransform(pts1, pts2)
image = cv2.warpAffine(image, M, shape_size[::-1], borderMode=cv2.BORDER_REFLECT_101)
dx = gaussian_filter((random_state.rand(*shape) * 2 - 1), sigma) * alpha
dy = gaussian_filter((random_state.rand(*shape) * 2 - 1), sigma) * alpha
dz = np.zeros_like(dx)
x, y, z = np.meshgrid(np.arange(shape[1]), np.arange(shape[0]), np.arange(shape[2]))
indices = np.reshape(y+dy, (-1, 1)), np.reshape(x+dx,(-1,1)), np.reshape(z+dz,(-1,1))
return map_coordinates(image, indices, order=1, mode='reflect').reshape(shape)
def gaussian_filter(X, sigma):
filter_shape = 2 * np.ceil(2 * sigma) + 1
x = np.arange(-filter_shape/2, filter_shape/2+1)
gauss_filter = np.exp(-x**2/(2*sigma**2))
gauss_filter /= np.sum(gauss_filter)
return np.apply_along_axis(lambda m: np.convolve(m, gauss_filter, mode='valid'), 0, X)
def map_coordinates(X, indices, order=1, mode='reflect'):
output_shape = indices[0].shape
indices = np.reshape(indices, (len(X.shape), -1))
indices = np.concatenate((indices, np.zeros((len(X.shape)-indices.shape[0], indices.shape[1]))))
X = np.pad(X, ((1,1),(1,1),(1,1)), mode=mode)
output = np.zeros(output_shape)
for i, coord in enumerate(indices):
output += X[tuple([coord + 1])] * _bspline_kernel(coord - indices[i], order=order)
return output
def _bspline_kernel(x, order):
if order == 0:
return np.where(np.abs(x) < 1, 1, 0)
elif order == 1:
return np.where(np.abs(x) < 0.5, 0.75 - x**2, np.where(np.abs(x) < 1.5, 0.5 * (1.5 - np.abs(x))**2, 0))
elif order == 2:
return np.where(np.abs(x) < 1, 1/3 - x**2 + 0.5*np.abs(x)**3, np.where(np.abs(x) < 2, 1/6*(2-x)**3, 0))
elif order == 3:
return np.where(np.abs(x) < 0.5, 2/3 - 4/3*x**2 + 0.5*np.abs(x)**3, np.where(np.abs(x) < 1.5, 1/12*(2-x)**3 - 2/3*(1-x)**3 + 0.75*(1-np.abs(x))**3, np.where(np.abs(x) < 2.5, 1/12*(2-x)**3 + 2/3*(1-x)**3 + 0.75*(1+np.abs(x))**3 - 2*(2+np.abs(x))**3/3/np.abs(x)**3, 0)))
if __name__ == '__main__':
path = 'path/to/images/'
alpha = 2000
sigma = 20
alpha_affine = 50
for filename in os.listdir(path):
img = cv2.imread(os.path.join(path, filename))
img = elastic_transform(img, alpha, sigma, alpha_affine)
cv2.imwrite(os.path.join(path, 'elastic_' + filename), img)
```
仿射变换:
```python
import cv2
import numpy as np
import os
def affine_transform(image, alpha_affine, random_state=None):
if random_state is None:
random_state = np.random.RandomState(None)
shape = image.shape
shape_size = shape[:2]
center_square = np.float32(shape_size) // 2
square_size = min(shape_size) // 3
pts1 = np.float32([center_square + square_size, [center_square[0]+square_size, center_square[1]-square_size], center_square - square_size])
pts2 = pts1 + random_state.uniform(-alpha_affine, alpha_affine, size=pts1.shape).astype(np.float32)
M = cv2.getAffineTransform(pts1, pts2)
image = cv2.warpAffine(image, M, shape_size[::-1], borderMode=cv2.BORDER_REFLECT_101)
return image
if __name__ == '__main__':
path = 'path/to/images/'
alpha_affine = 50
for filename in os.listdir(path):
img = cv2.imread(os.path.join(path, filename))
img = affine_transform(img, alpha_affine)
cv2.imwrite(os.path.join(path, 'affine_' + filename), img)
```
旋转变换:
```python
import cv2
import numpy as np
import os
def rotate_transform(image, angle, scale=1.0):
(h, w) = image.shape[:2]
center = (w / 2, h / 2)
M = cv2.getRotationMatrix2D(center, angle, scale)
rotated = cv2.warpAffine(image, M, (w, h))
return rotated
if __name__ == '__main__':
path = 'path/to/images/'
angle = 30
for filename in os.listdir(path):
img = cv2.imread(os.path.join(path, filename))
img = rotate_transform(img, angle)
cv2.imwrite(os.path.join(path, 'rotate_' + filename), img)
```
相关推荐
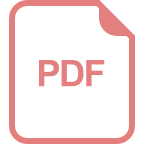
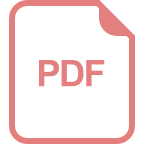
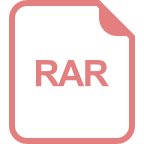














