写一个程序计算平面上两点之间的距离
时间: 2024-09-09 22:09:47 浏览: 64
当然可以。在编程中,计算平面上两点之间的距离通常使用欧几里得距离公式。这里是一个简单的Python示例:
```python
import math
def distance(point1, point2):
# 点的坐标通常表示为(x, y)
x1, y1 = point1
x2, y2 = point2
# 欧氏距离公式: sqrt((x2-x1)^2 + (y2-y1)^2)
return math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
# 使用函数
point_a = (0, 0) # (x, y)形式
point_b = (3, 4) # 另一点的坐标
distance_between_points = distance(point_a, point_b)
print(f"两点 {point_a} 和 {point_b} 之间的距离是: {distance_between_points}")
```
在这个例子中,`point1`和`point2`分别代表两个点的坐标,函数返回它们之间的直线距离。
相关问题
设计一个c++程序,利用友元函数计算平面上两点之间的距离
可以设计一个Point类表示平面上的点,并在类中定义一个友元函数来计算两点之间的距离。具体实现如下:
```c++
#include <iostream>
#include <cmath> // 引入数学函数库
using namespace std;
class Point {
private:
double x, y; // 表示平面上的点的 x 和 y 坐标
public:
Point(double _x, double _y) : x(_x), y(_y) {}
friend double distance(const Point& p1, const Point& p2); // 友元函数声明
};
double distance(const Point& p1, const Point& p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
return sqrt(dx*dx + dy*dy); // 计算两点之间的距离
}
int main() {
Point p1(1, 2);
Point p2(3, 4);
cout << "The distance between p1 and p2 is: " << distance(p1, p2) << endl;
return 0;
}
```
在上述程序中,Point 类中定义了一个友元函数 `distance`,该函数计算两个点之间的距离。在 `main` 函数中,我们创建了两个点 `p1` 和 `p2`,并调用 `distance` 函数来计算它们之间的距离。
计算平面上两点间的距离c语言
计算平面上两点间的距离的公式为:
distance = sqrt((x2 - x1)*(x2 - x1) + (y2 - y1)*(y2 - y1))
其中,(x1, y1) 和 (x2, y2) 分别代表两个点的坐标。
以下是一个简单的 C 代码实现:
```c
#include <stdio.h>
#include <math.h>
int main() {
int x1, y1, x2, y2;
double distance;
printf("Enter the coordinates of point 1: ");
scanf("%d %d", &x1, &y1);
printf("Enter the coordinates of point 2: ");
scanf("%d %d", &x2, &y2);
distance = sqrt((x2 - x1)*(x2 - x1) + (y2 - y1)*(y2 - y1));
printf("Distance between the two points is: %lf\n", distance);
return 0;
}
```
在这个程序中,我们使用 `scanf()` 函数从用户那里获取两个点的坐标,然后使用 `sqrt()` 函数计算距离,并使用 `printf()` 函数显示结果。
阅读全文
相关推荐
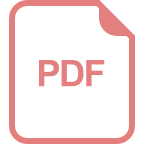
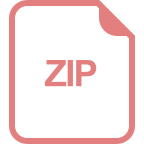
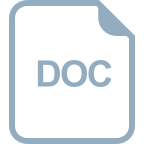













