用torch实现2层GCN
时间: 2023-03-14 19:03:17 浏览: 92
我不是很熟悉用Torch实现二层GCN,但是我可以尝试为您提供一些建议。首先,您可以使用PyTorch库中提供的GCN模块,它可以帮助您构建有效的GCN架构。其次,您可以参考其他开发者实现的GCN代码,以获得有关如何构建2层GCN的更多信息。祝您好运!
相关问题
torch实现空间注意力GCN
要PyTorch实现带有空间注意力机制GCN(Graph Convolutional Network),您可以按照以下步骤进行操作:
1. 导入所需的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import MessagePassing
from torch_geometric.utils import add_self_loops, degree
```
2. 定义一个自定义的GCNConv类,继承自MessagePassing类,并实现消息传递和聚合方法:
```python
class GCNConv(MessagePassing):
def __init__(self, in_channels, out_channels):
super(GCNConv, self).__init__(aggr='add')
self.lin = nn.Linear(in_channels, out_channels)
def forward(self, x, edge_index):
# 添加自环边
edge_index, _ = add_self_loops(edge_index, num_nodes=x.size(0))
# 计算归一化的度
row, col = edge_index
deg = degree(col, x.size(0), dtype=x.dtype)
deg_inv_sqrt = deg.pow(-0.5)
norm = deg_inv_sqrt[row] * deg_inv_sqrt[col]
# 转换特征向量
x = self.lin(x)
return self.propagate(edge_index, size=(x.size(0), x.size(0)), x=x, norm=norm)
def message(self, x_j, norm):
# 乘以归一化系数
return norm.view(-1, 1) * x_j
```
3. 定义包含空间注意力机制的GCN模型:
```python
class GCNWithAttention(nn.Module):
def __init__(self, in_channels, hidden_channels, out_channels):
super(GCNWithAttention, self).__init__()
self.conv1 = GCNConv(in_channels, hidden_channels)
self.att = nn.Linear(hidden_channels, 1)
self.conv2 = GCNConv(hidden_channels, out_channels)
def forward(self, x, edge_index):
x = F.relu(self.conv1(x, edge_index))
x = F.dropout(x, training=self.training)
# 计算节点的空间注意力权重
att_weights = torch.sigmoid(self.att(x))
# 应用空间注意力权重
x = x * att_weights
x = self.conv2(x, edge_index)
return x
```
这样,您就可以使用GCNWithAttention模型来进行图数据的处理,其中空间注意力机制被应用于增强节点之间的信息传递和聚合过程。请注意,这只是一个简单的示例,您可以根据需要进行修改和扩展。
pytorch实现两层gcn
以下是 PyTorch 实现两层 GCN 的代码示例:
```python
import torch
import torch.nn as nn
class GCNLayer(nn.Module):
def __init__(self, in_features, out_features):
super(GCNLayer, self).__init__()
self.linear = nn.Linear(in_features, out_features)
self.relu = nn.ReLU()
def forward(self, x, adj):
x = self.linear(x)
x = torch.matmul(adj, x)
x = self.relu(x)
return x
class GCN(nn.Module):
def __init__(self, nfeat, nhid, nclass):
super(GCN, self).__init__()
self.layer1 = GCNLayer(nfeat, nhid)
self.layer2 = GCNLayer(nhid, nclass)
def forward(self, x, adj):
x = self.layer1(x, adj)
x = self.layer2(x, adj)
return x
```
其中,`GCNLayer` 是一个基本的 GCN 层,它接受输入特征、邻接矩阵作为输入,并输出 GCN 层处理后的结果。`GCN` 则是由两个 `GCNLayer` 组成的两层 GCN 网络,它接收输入特征和邻接矩阵,并输出 GCN 处理后的结果。可以根据需要调整输入特征的维度、隐藏层的维度和输出层的维度来适应不同的任务。
阅读全文
相关推荐
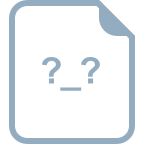
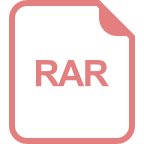

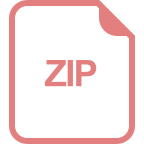











