请用C++写一个贪吃蛇小程序
时间: 2024-01-05 17:04:48 浏览: 126
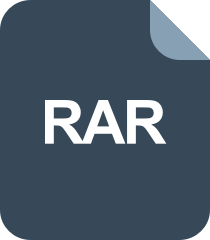
C++实现-贪吃蛇小游戏-贪吃蛇
好的,以下是一个简单的贪吃蛇小程序的 C++ 实现:
```cpp
#include <iostream>
#include <conio.h>
#include <stdlib.h>
#include <time.h>
using namespace std;
const int width = 20; // 地图宽度
const int height = 20; // 地图高度
class Snake {
public:
Snake(int x, int y, int len = 3) {
head_x = x;
head_y = y;
length = len;
for (int i = 0; i < length; i++) {
body_x[i] = head_x - i;
body_y[i] = head_y;
}
}
void move(int dx, int dy) {
// 移动身体
for (int i = length - 1; i > 0; i--) {
body_x[i] = body_x[i - 1];
body_y[i] = body_y[i - 1];
}
// 移动头部
head_x += dx;
head_y += dy;
body_x[0] = head_x;
body_y[0] = head_y;
}
bool is_dead() {
// 判断是否撞墙
if (head_x < 0 || head_x >= width || head_y < 0 || head_y >= height) {
return true;
}
// 判断是否撞到自己
for (int i = 1; i < length; i++) {
if (head_x == body_x[i] && head_y == body_y[i]) {
return true;
}
}
return false;
}
void grow() {
length++;
body_x[length - 1] = body_x[length - 2];
body_y[length - 1] = body_y[length - 2];
}
void draw() {
for (int i = 0; i < length; i++) {
cout << "O";
}
cout << endl;
}
int head_x, head_y; // 头部坐标
int body_x[100], body_y[100]; // 身体坐标
int length; // 蛇的长度
};
class Food {
public:
Food() {
x = rand() % width;
y = rand() % height;
}
void respawn() {
x = rand() % width;
y = rand() % height;
}
void draw() {
cout << "F" << endl;
}
int x, y; // 食物坐标
};
class Game {
public:
Game() {
srand(time(NULL));
score = 0;
snake = new Snake(width / 2, height / 2);
food = new Food();
}
~Game() {
delete snake;
delete food;
}
void run() {
while (true) {
system("cls"); // 清屏
draw_map(); // 绘制地图
snake->draw(); // 绘制蛇
food->draw(); // 绘制食物
if (snake->head_x == food->x && snake->head_y == food->y) {
snake->grow(); // 吃到食物,蛇变长
food->respawn(); // 食物重生
score++; // 得分加一
}
if (snake->is_dead()) {
cout << "Game over! Score: " << score << endl;
break;
}
if (_kbhit()) {
char ch = _getch();
switch (ch) {
case 'w':
snake->move(0, -1); // 向上移动
break;
case 's':
snake->move(0, 1); // 向下移动
break;
case 'a':
snake->move(-1, 0); // 向左移动
break;
case 'd':
snake->move(1, 0); // 向右移动
break;
}
}
else {
snake->move(0, 0); // 无操作,蛇保持原地
}
Sleep(100); // 暂停一段时间
}
}
void draw_map() {
for (int i = 0; i <= width + 1; i++) {
cout << "#";
}
cout << endl;
for (int j = 0; j < height; j++) {
cout << "#";
for (int i = 0; i < width; i++) {
if (i == snake->head_x && j == snake->head_y) {
cout << "H"; // 绘制蛇头
}
else if (i == food->x && j == food->y) {
cout << "F"; // 绘制食物
}
else {
bool is_body = false;
for (int k = 0; k < snake->length; k++) {
if (i == snake->body_x[k] && j == snake->body_y[k]) {
cout << "O"; // 绘制蛇身
is_body = true;
break;
}
}
if (!is_body) {
cout << " "; // 绘制空格
}
}
}
cout << "#" << endl;
}
for (int i = 0; i <= width + 1; i++) {
cout << "#";
}
cout << endl;
}
int score; // 得分
Snake* snake; // 蛇
Food* food; // 食物
};
int main() {
Game game;
game.run();
return 0;
}
```
该程序在 Windows 系统下编译运行,使用了 conio.h 头文件中的 _kbhit 和 _getch 函数来实现键盘输入检测。游戏的规则简单:通过 w、s、a、d 控制蛇的移动,吃到食物会得分并使蛇变长,撞墙或撞到自己则游戏结束。
阅读全文
相关推荐
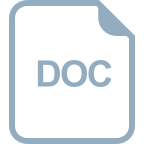
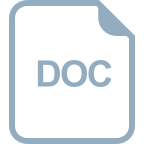



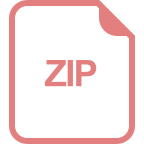
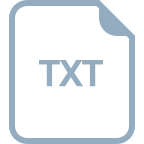
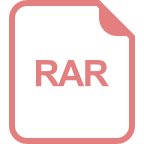
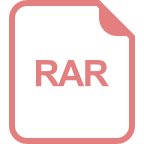
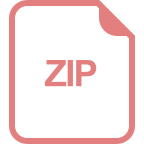




