c++ opencv 对数变换
时间: 2023-11-15 18:04:55 浏览: 60
C++ OpenCV中的对数变换可以通过以下步骤实现:
1. 读取图像并将其转换为灰度图像。
2. 对图像进行归一化处理,使其像素值在0到255之间。
3. 对图像进行傅里叶变换,并将其中心化。
4. 计算傅里叶变换的幅度谱,并将其映射到0到255之间。
5. 对幅度谱进行对数变换,并将其映射到0到255之间。
6. 显示原始图像、傅里叶变换的幅度谱和对数变换后的幅度谱。
以下是C++ OpenCV中实现对数变换的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
Mat img = imread("image.jpg", IMREAD_GRAYSCALE);
if (img.empty())
{
cout << "Could not read the image" << endl;
return -1;
}
Mat img_norm;
normalize(img, img_norm, 0, 255, NORM_MINMAX);
Mat img_fft;
dft(img_norm, img_fft);
Mat img_fft_shift;
fftshift(img_fft, img_fft_shift);
Mat img_amp;
magnitude(img_fft_shift, img_amp);
Mat img_amp_norm;
normalize(img_amp, img_amp_norm, 0, 255, NORM_MINMAX);
Mat img_amp_log;
img_amp_log = Mat::zeros(img_amp.size(), CV_32F);
for (int i = 0; i < img_amp.rows; i++)
{
for (int j = 0; j < img_amp.cols; j++)
{
img_amp_log.at<float>(i, j) = log(1 + img_amp_norm.at<float>(i, j));
}
}
Mat img_amp_log_norm;
normalize(img_amp_log, img_amp_log_norm, 0, 255, NORM_MINMAX);
Mat img_show;
hconcat(img_norm, img_amp_norm, img_show);
hconcat(img_show, img_amp_log_norm, img_show);
imshow("Logarithmic Transformation", img_show);
waitKey(0);
return 0;
}
```
相关推荐
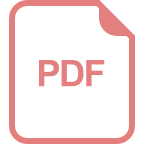
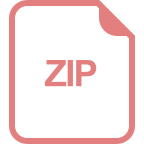














