利用仿射变换加密解密的C++程序
时间: 2024-10-22 19:15:32 浏览: 62
仿射变换是一种简单的数字图像处理技术,在密码学中也被用于对数据进行轻度混淆,特别是在教育和教学环境中作为基础加密算法演示。在C++中,我们可以创建一个简单的仿射加密程序,其核心步骤包括选择两个关键值(常数a和b),将每个像素点通过公式 (ax + b) % 256 进行加密,同样解密则通过同样的公式但用逆操作 (x * a_inverse - b) % 256。
以下是一个简化的C++代码示例:
```cpp
#include <iostream>
#include <vector>
// 定义仿射矩阵
const int a = 7; // 加密系数
const int b = 13; // 偏移量
// 仿射加密函数
std::vector<int> encrypt(const std::vector<int>& pixels) {
std::vector<int> encrypted;
for (int pixel : pixels) {
encrypted.push_back((a * pixel + b) % 256);
}
return encrypted;
}
// 解密函数,需要计算a_inv(a的逆元)
int a_inv = modInverse(a, 256); // 可能需要一个自定义的modInverse函数
std::vector<int> decrypt(const std::vector<int>& encrypted) {
std::vector<int> decrypted;
for (int enc : encrypted) {
decrypted.push_back(((enc - b) * a_inv) % 256);
}
return decrypted;
}
// 模运算逆元函数(这里简化版本,实际实现可能更复杂)
int modInverse(int a, int m) {
int x = 0, y = 1;
while (y != 0) {
int q = a / m;
int t = m;
m = a % m;
a = t;
t = x;
x = y;
y = t - q * y;
}
return x;
}
int main() {
std::vector<int> original = {1, 2, 3, 4, 5}; // 示例原图像素
std::vector<int> encrypted = encrypt(original);
std::cout << "Original pixels: ";
for (int p : original) {
std::cout << p << " ";
}
std::cout << "\nEncrypted pixels: ";
for (int p : encrypted) {
std::cout << p << " ";
}
std::vector<int> decrypted = decrypt(encrypted);
std::cout << "\nDecrypted pixels: ";
for (int p : decrypted) {
std++.cout << p << " ";
}
return 0;
}
```
注意:这个例子只展示了加密和解密的基本流程,真正的应用中你需要考虑如何处理边界条件、边缘情况以及可能的数据完整性检查等。
阅读全文
相关推荐
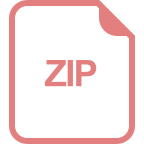

















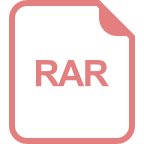