用c语言编写(1)已知f为单链表的表头指针,链表中存储的都是整型数据,试写出实现下列运算的递归算法,要求只需要写出具体函数即可: ①求链表中的最大整数; ②求链表中的节点个数; ③求所有整数的平均值。
时间: 2024-12-11 19:19:31 浏览: 23
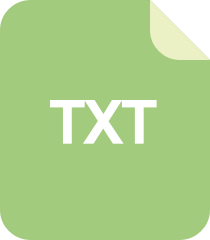
链表的C语言实现之单链表的查找运算.txt
在C语言中,我们可以使用递归来遍历链表并实现这些操作。由于递归涉及返回自身来处理更小的问题,这里我会给出三个函数的具体实现:
1. **求链表最大整数** (max_in_list):
```c
#include <stdio.h>
#include <limits.h>
struct Node {
int data;
struct Node* next;
};
int max_in_list(struct Node* head) {
if (head == NULL || head->next == NULL) { // 链表为空或只有一个元素
return head->data;
} else {
int max_here = head->data; // 当前节点的最大值
int next_max = max_in_list(head->next); // 递归查找下一个节点的最大值
return max_here > next_max ? max_here : next_max; // 返回较大者
}
}
```
2. **求链表节点个数** (count_nodes):
```c
int count_nodes(struct Node* head) {
if (head == NULL) { // 链表为空
return 0;
} else {
return 1 + count_nodes(head->next); // 递归加一,直到遍历到尾部
}
}
```
3. **求链表平均值** (average_of_list):
```c
double average_of_list(struct Node* head) {
if (head == NULL) { // 链表为空
return 0.0;
} else {
double sum = head->data; // 初始化总和
int count = 1;
struct Node* current = head->next; // 指向第二个节点
while (current != NULL) {
sum += current->data;
count++;
current = current->next;
}
return sum / count; // 除以节点数得到平均值
}
}
```
阅读全文
相关推荐
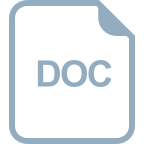
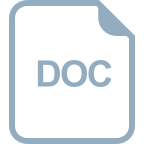











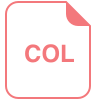
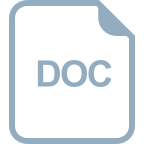
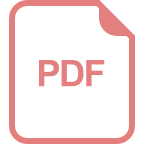
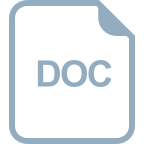
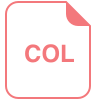