C++定义一个字符串类,重载的运算符包括:=、+、+=、>、<、>=、<=、==、!=。其中,除=、+=运算符用成员函数定义外、其余的运算符要求用友元方式定义完整代码
时间: 2024-10-27 07:09:42 浏览: 46
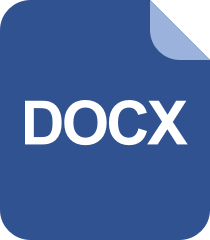
C++中各种重载运算符程序
在C++中,为了定义一个支持上述运算符的字符串类,我们通常需要创建一个类(例如String),并使其成为流插入和提取运算符的友元。同时,我们将赋值(=)和连接(+=)操作作为成员函数实现,因为它们涉及对象自身的状态改变。其他比较运算符(>, <, >=, <=, ==, !=)由于不需要修改对象本身,所以可以定义为友元函数。
下面是一个简单的示例:
```cpp
#include <iostream>
#include <string>
class String {
public:
// 初始化一个空字符串
String() : value("") {}
// 赋值运算符 (成员函数)
String& operator=(const std::string& other) {
if (this != &other) {
value = other;
}
return *this;
}
// 连接运算符 (+=) 成员函数
String& operator+=(const std::string& other) {
value += other;
return *this;
}
// 返回字符串内容
friend std::ostream& operator<<(std::ostream& out, const String& str) {
out << str.value;
return out;
}
// 友元函数实现比较运算符
friend bool operator>(const String& a, const String& b) { return a.value > b.value; }
friend bool operator<(const String& a, const String& b) { return a.value < b.value; }
friend bool operator>=(const String& a, const String& b) { return a.value >= b.value; }
friend bool operator<=(const String& a, const String& b) { return a.value <= b.value; }
friend bool operator==(const String& a, const String& b) { return a.value == b.value; }
friend bool operator!=(const String& a, const String& b) { return a.value != b.value; }
private:
std::string value;
};
int main() {
String s1("Hello"), s2("World");
s1 = "C++";
s1 += "!";
std::cout << "s1: " << s1 << "\n"; // 输出 "C++!"
if (s1 > s2) {
std::cout << "s1 is greater than s2\n";
} else if (s1 < s2) {
std::cout << "s1 is less than s2\n";
} else {
std::cout << "s1 and s2 are equal\n";
}
return 0;
}
```
阅读全文
相关推荐
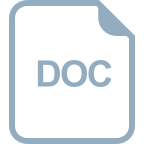
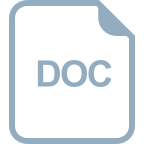










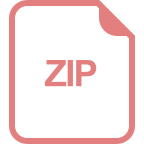
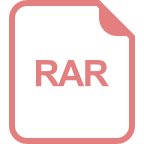



