C# Windows11API 实现蓝牙链接通信
时间: 2023-07-12 22:28:40 浏览: 134
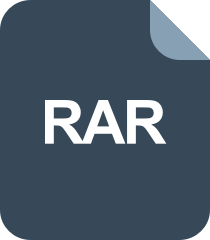
C# 关于蓝牙的通信开发

要使用C# Windows 11 API实现蓝牙连接通信,可以按照以下步骤进行:
1. 确认蓝牙适配器已经连接到计算机上,并且已经打开。
2. 使用Windows.Devices.Bluetooth命名空间中的BluetoothLEDevice.FromBluetoothAddressAsync方法获取蓝牙设备对象。
3. 使用Windows.Devices.Enumeration命名空间中的DeviceInformation.FindAllAsync方法查找设备服务。
4. 使用Windows.Devices.Bluetooth.GenericAttributeProfile命名空间中的GattDeviceService.GetCharacteristicsAsync方法获取设备特性。
5. 使用Windows.Devices.Bluetooth.GenericAttributeProfile命名空间中的GattCharacteristic.ValueChanged事件处理程序接收设备发送的数据。
6. 使用Windows.Devices.Bluetooth.GenericAttributeProfile命名空间中的GattCharacteristic.WriteValueAsync方法写入数据。
示例代码如下:
```csharp
using Windows.Devices.Bluetooth;
using Windows.Devices.Enumeration;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
using System;
using System.Threading.Tasks;
namespace BluetoothCommunication
{
class Program
{
private static GattCharacteristic _characteristic;
static async Task Main(string[] args)
{
var deviceSelector = BluetoothLEDevice.GetDeviceSelectorFromConnectionStatus(BluetoothConnectionStatus.Connected);
var deviceInformationCollection = await DeviceInformation.FindAllAsync(deviceSelector);
if (deviceInformationCollection.Count > 0)
{
var bluetoothLeDevice = await BluetoothLEDevice.FromIdAsync(deviceInformationCollection[0].Id);
if (bluetoothLeDevice != null)
{
var servicesResult = await bluetoothLeDevice.GetGattServicesAsync();
if (servicesResult.Status == GattCommunicationStatus.Success)
{
foreach (var service in servicesResult.Services)
{
var characteristicsResult = await service.GetCharacteristicsAsync();
if (characteristicsResult.Status == GattCommunicationStatus.Success)
{
foreach (var characteristic in characteristicsResult.Characteristics)
{
if (characteristic.CharacteristicProperties.HasFlag(GattCharacteristicProperties.Write))
{
_characteristic = characteristic;
characteristic.ValueChanged += Characteristic_ValueChanged;
}
}
}
}
}
}
}
}
private static async void Characteristic_ValueChanged(GattCharacteristic sender, GattValueChangedEventArgs args)
{
var reader = Windows.Storage.Streams.DataReader.FromBuffer(args.CharacteristicValue);
var data = reader.ReadString(args.CharacteristicValue.Length);
Console.WriteLine("Received data: " + data);
}
private static async Task SendData(string data)
{
var writer = new Windows.Storage.Streams.DataWriter();
writer.WriteString(data);
var result = await _characteristic.WriteValueAsync(writer.DetachBuffer());
if (result == GattCommunicationStatus.Success)
{
Console.WriteLine("Data written successfully");
}
}
}
}
```
需要注意的是,要使用Windows 11 API实现蓝牙连接通信需要在Windows 11操作系统中运行,并且需要保证设备支持BLE通信。
阅读全文
相关推荐












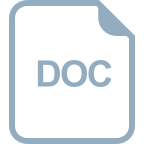
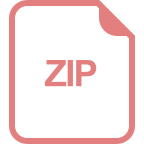
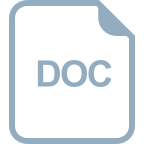
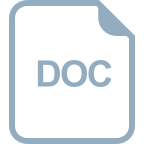