用C++写一个MODBUS TCP的程序
时间: 2023-10-13 15:06:39 浏览: 37
好的,以下是一个简单的 MODBUS TCP 的程序示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#define SERVER_IP "127.0.0.1"
#define SERVER_PORT 502
int main(int argc, char *argv[]) {
int sock;
struct sockaddr_in server;
char message[1024], server_reply[1024];
uint16_t tx_buffer[256], rx_buffer[256];
int i, j, tx_len, rx_len, num_regs;
// 创建 socket
sock = socket(AF_INET, SOCK_STREAM, 0);
if (sock == -1) {
printf("Could not create socket\n");
return 1;
}
server.sin_addr.s_addr = inet_addr(SERVER_IP);
server.sin_family = AF_INET;
server.sin_port = htons(SERVER_PORT);
// 连接服务器
if (connect(sock, (struct sockaddr *)&server, sizeof(server)) < 0) {
printf("Connect failed\n");
return 1;
}
// 构造请求帧
tx_len = 0;
tx_buffer[tx_len++] = htons(0x0001); // 事务标识符
tx_buffer[tx_len++] = htons(0x0000); // 协议标识符
tx_buffer[tx_len++] = htons(0x0006); // 长度字段
tx_buffer[tx_len++] = htons(0x0000); // 单元标识符
tx_buffer[tx_len++] = htons(0x0003); // 功能码
tx_buffer[tx_len++] = htons(0x0000); // 起始地址
tx_buffer[tx_len++] = htons(0x0001); // 寄存器数量
// 发送请求帧
if (send(sock, tx_buffer, tx_len * sizeof(uint16_t), 0) != tx_len * sizeof(uint16_t)) {
printf("Send failed\n");
return 1;
}
// 接收响应帧
rx_len = recv(sock, rx_buffer, sizeof(rx_buffer), 0);
if (rx_len < 0) {
printf("Receive failed\n");
return 1;
}
// 解析响应帧
num_regs = rx_buffer[2] / sizeof(uint16_t);
printf("Received %d registers:\n", num_regs);
for (i = 0; i < num_regs; i++) {
j = i * sizeof(uint16_t) + 3;
printf("%04X\n", ntohs(rx_buffer[j]));
}
close(sock);
return 0;
}
```
注意:这只是一个简单的示例,实际使用中需要根据具体的设备和寄存器地址进行修改。
相关推荐
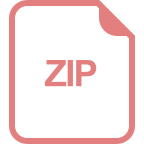














