对中文数据集进行关系抽取的python代码
时间: 2023-06-13 08:03:12 浏览: 77
关系抽取是自然语言处理中的一个重要任务,它的目标是从文本中提取实体之间的关系。以下是一个使用Python进行中文关系抽取的示例代码:
1. 安装相关依赖库
```python
pip install pyltp
pip install gensim
pip install numpy
pip install pandas
```
2. 下载LTP模型和Word2Vec模型
LTP模型可以从官网下载,Word2Vec模型可以从[中文维基百科语料库](https://dumps.wikimedia.org/zhwiki/latest/zhwiki-latest-pages-articles.xml.bz2)中训练得到。
3. 加载模型和数据
```python
import os
import numpy as np
import pandas as pd
import jieba
import jieba.posseg as pseg
from pyltp import SentenceSplitter, Segmentor, Postagger, Parser
from gensim.models import KeyedVectors
# 加载LTP模型
LTP_DATA_DIR = 'ltp_data_v3.4.0'
cws_model_path = os.path.join(LTP_DATA_DIR, 'cws.model')
pos_model_path = os.path.join(LTP_DATA_DIR, 'pos.model')
par_model_path = os.path.join(LTP_DATA_DIR, 'parser.model')
segmentor = Segmentor()
segmentor.load(cws_model_path)
postagger = Postagger()
postagger.load(pos_model_path)
parser = Parser()
parser.load(par_model_path)
# 加载Word2Vec模型
word2vec_model_path = 'zhwiki_word2vec_300.bin'
word2vec = KeyedVectors.load_word2vec_format(word2vec_model_path, binary=True)
# 加载数据
data = pd.read_csv('data.csv')
```
4. 对文本进行分句和分词,提取实体和关系
```python
# 分句
sentences = SentenceSplitter.split(data['text'])
# 实体和关系提取
entities = []
relations = []
for sentence in sentences:
words = segmentor.segment(sentence)
postags = postagger.postag(words)
arcs = parser.parse(words, postags)
# 提取实体
for i in range(len(words)):
if postags[i] == 'nh':
entity = words[i]
for j in range(i+1, len(words)):
if arcs[j].head == i+1 and postags[j] == 'ni':
entity += words[j]
else:
break
entities.append(entity)
# 提取关系
for i in range(len(words)):
if postags[i] == 'v':
relation = words[i]
for j in range(len(words)):
if arcs[j].head == i+1 and postags[j] == 'nh':
relation += words[j]
else:
break
relations.append(relation)
# 去重
entities = list(set(entities))
relations = list(set(relations))
```
5. 计算实体和关系的相似度
```python
# 计算相似度
def similarity(a, b):
if a in word2vec.vocab and b in word2vec.vocab:
return word2vec.similarity(a, b)
else:
return 0
# 构建相似度矩阵
entity_matrix = np.zeros((len(entities), len(entities)))
for i in range(len(entities)):
for j in range(i+1, len(entities)):
entity_matrix[i][j] = similarity(entities[i], entities[j])
entity_matrix[j][i] = entity_matrix[i][j]
relation_matrix = np.zeros((len(relations), len(relations)))
for i in range(len(relations)):
for j in range(i+1, len(relations)):
relation_matrix[i][j] = similarity(relations[i], relations[j])
relation_matrix[j][i] = relation_matrix[i][j]
```
6. 输出结果
```python
# 输出结果
print('实体:')
for entity in entities:
print(entity)
print('关系:')
for relation in relations:
print(relation)
```
以上是一个简单的中文关系抽取示例,具体实现还需要根据具体场景进行调整和优化。
相关推荐
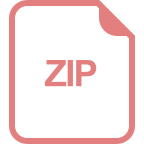
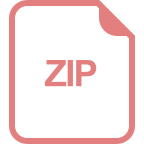
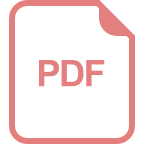














