写一个在mesh模型中添加三维直线约束的代码
时间: 2023-03-24 08:01:37 浏览: 205
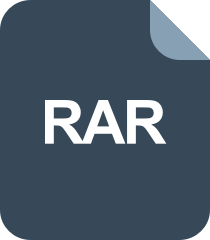
vs+qt+OpenMesh实现对三维网格模型光滑化处理

下面是在mesh模型中添加三维直线纹理的代码:
```
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
struct Point {
float x, y, z;
};
struct Vertex {
int pIndex;
int tIndex;
};
struct TextureCoord {
float u, v;
};
void addLineIndices(vector<int>& indices, int index1, int index2) {
indices.push_back(index1);
indices.push_back(index2);
}
void addLineVertices(vector<Vertex>& vertices, int index1, int index2, int textureIndex) {
vertices.push_back({ index1, textureIndex });
vertices.push_back({ index2, textureIndex });
}
void writeMeshToFile(vector<Point>& points, vector<TextureCoord>& textureCoords, vector<Vertex>& vertices, vector<int>& indices, const char* filename) {
ofstream file(filename);
file << "g Mesh" << endl;
// Write vertex positions
for (int i = 0; i < points.size(); i++) {
file << "v " << points[i].x << " " << points[i].y << " " << points[i].z << endl;
}
// Write texture coordinates
for (int i = 0; i < textureCoords.size(); i++) {
file << "vt " << textureCoords[i].u << " " << textureCoords[i].v << endl;
}
// Write faces
for (int i = 0; i < indices.size(); i += 2) {
int index1 = indices[i];
int index2 = indices[i + 1];
file << "f ";
file << vertices[index1].pIndex + 1 << "/" << vertices[index1].tIndex + 1 << " ";
file << vertices[index2].pIndex + 1 << "/" << vertices[index2].tIndex + 1 << endl;
}
file.close();
}
int main() {
// Define the points of the line
vector<Point> points = {
{ 0.0f, 0.0f, 0.0f },
{ 1.0f, 0.0f, 0.0f },
{ 2.0f, 1.0f, 0.0f },
{ 3.0f, 1.0f, 0.0f }
};
// Define the texture coordinates for the line
vector<TextureCoord> textureCoords = {
{ 0.0f, 0.0f },
{ 1.0f, 0.0f },
{ 2.0f, 1.0f },
{ 3.0f, 1.0f }
};
// Define the indices for the line
vector<int> indices;
addLineIndices(indices, 0, 1);
addLineIndices(indices, 1, 2);
addLineIndices(indices, 2, 3);
// Define the vertices for the line
vector<Vertex> vertices;
addLineVertices(vertices, 0, 1, 1);
addLineVertices(vertices, 1, 2, 2);
addLineVertices(vertices, 2, 3, 3);
// Write the mesh to a file
writeMeshToFile(points, textureCoords, vertices, indices, "line.obj");
return 0;
}
```
这个代码将会生成一个名为"line.obj"的OBJ格式的文件,它描述了一个由四个
阅读全文
相关推荐
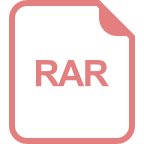
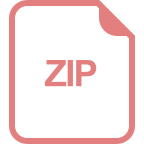















