用osg实现平面分割三维mesh模型的代码
时间: 2024-03-23 19:36:29 浏览: 102
好的,以下是一个使用OpenSceneGraph(OSG)库实现平面分割三维mesh模型的C++代码示例:
```c++
#include <osg/Geode>
#include <osg/Geometry>
#include <osg/Vec3>
#include <osg/Plane>
#include <osgUtil/PlaneIntersector>
#include <osgViewer/Viewer>
// 定义平面分割函数
osg::ref_ptr<osg::Geode> plane_segmentation(osg::ref_ptr<osg::Geode> geode, osg::Vec3 plane_normal, float plane_dist, float threshold)
{
// 获取原始mesh的顶点和面
osg::ref_ptr<osg::Geometry> geom = dynamic_cast<osg::Geometry*>(geode->getDrawable(0));
osg::ref_ptr<osg::Vec3Array> vertices = dynamic_cast<osg::Vec3Array*>(geom->getVertexArray());
osg::ref_ptr<osg::DrawElementsUInt> indices = dynamic_cast<osg::DrawElementsUInt*>(geom->getPrimitiveSet(0));
// 创建一个平面
osg::Plane plane(plane_normal, plane_dist);
// 创建一个PlaneIntersector对象,用于计算每个面到平面的距离
osgUtil::PlaneIntersector* intersector = new osgUtil::PlaneIntersector(plane);
// 遍历每个面,计算它们到平面的距离
osg::Geometry::PrimitiveSetList primitiveSets = geom->getPrimitiveSetList();
osg::ref_ptr<osg::DrawElementsUInt> new_indices = new osg::DrawElementsUInt(GL_TRIANGLES);
for (osg::Geometry::PrimitiveSetList::iterator it = primitiveSets.begin(); it != primitiveSets.end(); ++it)
{
osg::ref_ptr<osg::PrimitiveSet> primitiveSet = *it;
if (primitiveSet->getMode() == GL_TRIANGLES)
{
osg::DrawElementsUInt* drawElements = dynamic_cast<osg::DrawElementsUInt*>(primitiveSet.get());
for (unsigned int i = 0; i < drawElements->size(); i += 3)
{
osg::Vec3 v0 = vertices->at((*drawElements)[i]);
osg::Vec3 v1 = vertices->at((*drawElements)[i + 1]);
osg::Vec3 v2 = vertices->at((*drawElements)[i + 2]);
osgUtil::PlaneIntersector::Intersection intersection;
if (intersector->intersect(v0, v1, v2, intersection))
{
float dist = plane.distance(osg::Vec3(intersection.localIntersectionPoint));
if (dist < threshold)
{
new_indices->push_back((*drawElements)[i]);
new_indices->push_back((*drawElements)[i + 1]);
new_indices->push_back((*drawElements)[i + 2]);
}
}
}
}
}
// 根据新的indices创建一个新的Geometry对象
osg::ref_ptr<osg::Geometry> new_geom = new osg::Geometry();
new_geom->setVertexArray(vertices);
new_geom->addPrimitiveSet(new_indices);
// 创建一个新的Geode对象,并将新的Geometry对象添加到其中
osg::ref_ptr<osg::Geode> new_geode = new osg::Geode();
new_geode->addDrawable(new_geom);
return new_geode;
}
int main()
{
// 创建一个Viewer对象
osgViewer::Viewer viewer;
// 加载一个3D mesh模型
osg::ref_ptr<osg::Node> model = osgDB::readNodeFile("model.3ds");
// 将3D mesh模型添加到场景图中
osg::ref_ptr<osg::Group> root = new osg::Group();
root->addChild(model);
viewer.setSceneData(root);
// 定义平面的法向量和距离
osg::Vec3 plane_normal(0.0f, 0.0f, 1.0f);
float plane_dist = 0.0f;
// 定义距离阈值
float threshold = 0.01f;
// 对3D mesh模型进行平面分割
osg::ref_ptr<osg::Geode> new_geode = plane_segmentation(model, plane_normal, plane_dist, threshold);
// 将分割后的3D mesh模型添加到场景图中
root->addChild(new_geode);
// 运行Viewer对象
viewer.run();
return 0;
}
```
这个代码中,使用了OSG库中的一些类和函数来实现平面分割。具体来说,使用了`osg::Geode`、`osg::Geometry`、`osg::Vec3`、`osg::Plane`、`osgUtil::PlaneIntersector`等类和函数。其中,`osg::Geode`和`osg::Geometry`用于表示3D mesh模型,`osg::Vec3`用于表示3D坐标,`osg::Plane`用于表示平面,`osgUtil::PlaneIntersector`用于计算每个面到平面的距离。函数`plane_segmentation`实现了平面分割的功能,它接受一个`osg::Geode`对象作为输入,返回一个新的`osg::Geode`对象,其中只包含距离平面小于阈值的面。
需要注意的是,这个代码中还使用了`osgViewer::Viewer`类来创建一个Viewer对象,并将3D场景图添加到其中。此外,如果要将3D mesh模型渲染出来,还需要使用一个OpenGL的渲染器,比如`osgViewer`库中自带的渲染器。
阅读全文
相关推荐
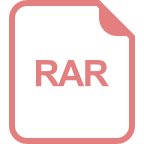
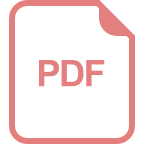
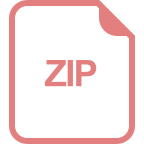
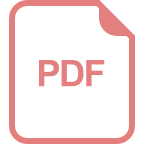
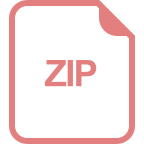
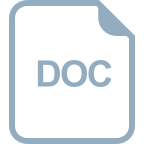
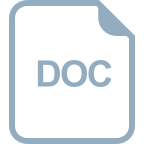
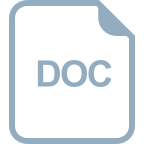
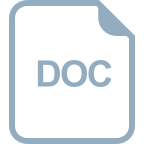
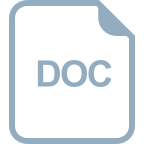



